So friends, today we will make such a CRUD operation which has already been made in the previous post. But this CRUD operation is unique. You may not have seen this type of “CRUD” operation elsewhere in Android Studio. Because of its unique UI design and easy method.
Today we will discuss CRUD (Create, Read, Update and Delete) operation with images. This means we will pick the image from the camera and gallery and store in SQLite database then we will display all data in listview also use additional UI design for additional design.
Perform operation
Insert data
Display data
Update data
Delete data
Search filter data
What is covered in this tutorial?
Picking image from camera and gallery and fit on ImageView
Inserting data into SQLite database with image.
Displaying image in ImageView.
Displaying data in a listview using a custom adapter.
Class, Activity and UI Design
DBmain : for managing SQLite database and tables.
MainActivity :default activity.
MainActivity2 : for displaying data logic.
Model: for storing data
activity_main: UI Design like EditText, ImageView and buttons.
Activity_main2: for creating Listview.
Singledata : single data file mean single item view.
Main_menu: To put a search filter on the action bar.
Now, we’ll be starting coding step by step.
First, we will create a local SQLite database class to store and manage the database and table.
Create class DBmain.java and extend SQLiteOpenHelper and override “onCreate” and “onUpdate” method.
The code of DBmain.java is given below.
DBmain.java
package com.example.myapplication;
import android.content.Context;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import androidx.annotation.Nullable;
public class DBmain extends SQLiteOpenHelper {
public static final String DBNAME="student.db";
public static final String TABLENAME="course";
public static final int VER=1;
public DBmain(@Nullable Context context) {
super(context, DBNAME, null, VER);
}
@Override
public void onCreate(SQLiteDatabase db) {
String query="create table "+TABLENAME+"(id integer primary key, name text, avatar blob)";
db.execSQL(query);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
String query="drop table if exists "+TABLENAME+"";
db.execSQL(query);
onCreate(db);
}
}
Now, we’ll make the user interface for input data like EditText, ImageView and buttons.
Go to activity_main.xml
Res ⇾ layout ⇾ activity_main.xml
Given below code of activity_main.xml.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<ImageView
android:layout_marginTop="10dp"
android:layout_gravity="center"
android:layout_width="150dp"
android:layout_height="150dp"
android:src="@mipmap/ic_launcher"
android:id="@+id/edtimage"/>
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Name"
android:inputType="text"
android:id="@+id/edtname"
android:layout_marginTop="10dp"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:layout_margin="10dp">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:id="@+id/btn_submit"
android:text="Submit"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/btn_edit"
android:visibility="gone"
android:text="Edit"
android:layout_weight="1"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Display"
android:id="@+id/btn_display"
android:layout_weight="1"/>
</LinearLayout>
</LinearLayout>
Go to MainActivtiy.java
app -> package name->java ->MainActivity.java
In MainActivity.java activity, we will give correct logic to pick image from camera and gallery and insert into SQLite database.
For cropping and loading image into imageview, we need dependency.
We’ll use the image cropping library.
Open build.gradle and add
api'com.theartofdev.edmodo:android-image-cropper:2.8.0'
and add permissions to manifest
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
And adding crop image activity
<activity android:name="com.theartofdev.edmodo.cropper.CropImageActivity" />
For displaying image into ImageView, we’ll use the below dependency in gradle.build
implementation'com.squareup.picasso:picasso:2.5.2' dependency.
See the below AndroidManifest file.
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.myapplication">
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.MyApplication">
<activity android:name=".MainActivity2"></activity>
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity android:name="com.theartofdev.edmodo.cropper.CropImageActivity" />
</application>
</manifest>
We already know about picking image from camera and gallery and inserting data into the SQLite database.
Given the below code for MainActivity.java.
MainActivity.java
package com.example.myapplication;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.annotation.RequiresApi;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.content.ContextCompat;
import android.Manifest;
import android.content.ContentValues;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.database.sqlite.SQLiteDatabase;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.drawable.BitmapDrawable;
import android.media.Image;
import android.net.Uri;
import android.os.Build;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.Toast;
import com.squareup.picasso.Picasso;
import com.theartofdev.edmodo.cropper.CropImage;
import java.io.ByteArrayOutputStream;
import static com.example.myapplication.DBmain.TABLENAME;
public class MainActivity extends AppCompatActivity {
DBmain dBmain;
SQLiteDatabase sqLiteDatabase;
EditText name;
Button submit, display, edit;
private ImageView avatar;
int id = 0;
public static final int CAMERA_REQUEST = 100;
public static final int STORAGE_REQUEST = 101;
String cameraPermission[];
String storagePermission[];
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
dBmain = new DBmain(this);
findid();
insertData();
editData();
avatar.setOnClickListener(new View.OnClickListener() {
@RequiresApi(api = Build.VERSION_CODES.M)
@Override
public void onClick(View v) {
int avatar = 0;
if (avatar == 0) {
if (!checkCameraPermission()) {
requstCameraPermission();
} else {
pickFromGallery();
}
} else if (avatar == 1) {
if (!checkStoragePermission()) {
requestStoragePermission();
} else {
pickFromGallery();
}
}
}
});
}
//edit data
private void editData() {
if (getIntent().getBundleExtra("userdata") != null) {
Bundle bundle = getIntent().getBundleExtra("userdata");
id = bundle.getInt("id");
name.setText(bundle.getString("name"));
//set image from database mean old image that you want ot update
byte[] bytes = bundle.getByteArray("avatar");
Bitmap bitmap = BitmapFactory.decodeByteArray(bytes, 0, bytes.length);
avatar.setImageBitmap(bitmap);
edit.setVisibility(View.VISIBLE);
submit.setVisibility(View.GONE);
}
}
// request storage permission
@RequiresApi(api = Build.VERSION_CODES.M)
private void requestStoragePermission() {
requestPermissions(storagePermission, STORAGE_REQUEST);
}
//check storage permissions
private boolean checkStoragePermission() {
boolean result = ContextCompat.checkSelfPermission(this, Manifest.permission.WRITE_EXTERNAL_STORAGE) == (PackageManager.PERMISSION_GRANTED);
return result;
}
// pick image from gallery
private void pickFromGallery() {
CropImage.activity().start(this);
}
// request camera permission
@RequiresApi(api = Build.VERSION_CODES.M)
private void requstCameraPermission() {
requestPermissions(cameraPermission, CAMERA_REQUEST);
}
// check camera permission
private boolean checkCameraPermission() {
boolean result = ContextCompat.checkSelfPermission(this, Manifest.permission.WRITE_EXTERNAL_STORAGE) == (PackageManager.PERMISSION_GRANTED);
boolean result1 = ContextCompat.checkSelfPermission(this, Manifest.permission.CAMERA) == (PackageManager.PERMISSION_GRANTED);
return result && result1;
}
// insert data into SQLite database
private void insertData() {
submit.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
ContentValues cv = new ContentValues();
cv.put("name", name.getText().toString());
cv.put("avatar", imageViewToBy(avatar));
sqLiteDatabase = dBmain.getWritableDatabase();
Long rec = sqLiteDatabase.insert("course", null, cv);
if (rec != null) {
Toast.makeText(MainActivity.this, "data inserted", Toast.LENGTH_SHORT).show();
name.setText("");
avatar.setImageResource(R.mipmap.ic_launcher);
} else {
Toast.makeText(MainActivity.this, "something wrong", Toast.LENGTH_SHORT).show();
}
}
});
display.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
startActivity(new Intent(MainActivity.this, MainActivity2.class));
}
});
edit.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
ContentValues cv = new ContentValues();
cv.put("name", name.getText().toString());
cv.put("avatar", imageViewToBy(avatar));
sqLiteDatabase = dBmain.getWritableDatabase();
long rededit = sqLiteDatabase.update(TABLENAME, cv, "id=" + id, null);
if (rededit != -1) {
Toast.makeText(MainActivity.this, "update successfully", Toast.LENGTH_SHORT).show();
submit.setVisibility(View.VISIBLE);
edit.setVisibility(View.GONE);
name.setText("");
} else {
Toast.makeText(MainActivity.this, "Data does not update", Toast.LENGTH_SHORT).show();
}
}
});
}
//convert image to byte array
public static byte[] imageViewToBy(ImageView avatar) {
Bitmap bitmap = ((BitmapDrawable) avatar.getDrawable()).getBitmap();
ByteArrayOutputStream stream = new ByteArrayOutputStream();
bitmap.compress(Bitmap.CompressFormat.JPEG, 50, stream);
byte[] bytes = stream.toByteArray();
return bytes;
}
//find id
private void findid() {
name = (EditText) findViewById(R.id.edtname);
avatar = (ImageView) findViewById(R.id.edtimage);
submit = (Button) findViewById(R.id.btn_submit);
display = (Button) findViewById(R.id.btn_display);
edit = (Button) findViewById(R.id.btn_edit);
}
// permission result
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
switch (requestCode) {
case CAMERA_REQUEST: {
if (grantResults.length > 0) {
boolean camera_accepted = grantResults[0] == PackageManager.PERMISSION_GRANTED;
boolean storage_accepted = grantResults[1] == PackageManager.PERMISSION_GRANTED;
if (camera_accepted && storage_accepted) {
pickFromGallery();
} else {
Toast.makeText(this, "enable camera and storage permission", Toast.LENGTH_SHORT).show();
}
}
}
break;
case STORAGE_REQUEST: {
if (grantResults.length > 0) {
boolean storage_accepted = grantResults[0] == PackageManager.PERMISSION_GRANTED;
if (storage_accepted) {
pickFromGallery();
} else {
Toast.makeText(this, "please enable storage permission", Toast.LENGTH_SHORT).show();
}
}
}
break;
}
}
// on activity result
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == CropImage.CROP_IMAGE_ACTIVITY_REQUEST_CODE) {
CropImage.ActivityResult result = CropImage.getActivityResult(data);
if (resultCode == RESULT_OK) {
Uri resultUri = result.getUri();
Picasso.with(this).load(resultUri).into(avatar);
}
}
}
}
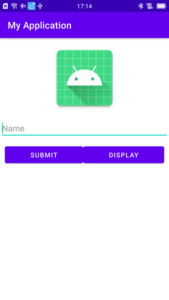
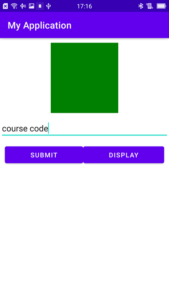
Now create a Model class and generate constructor and getter, setter methods.
The Model class code is given below.
Model.java
package com.example.myapplication;
public class Model {
private int id;
private String name;
private byte[]image;
public Model(int id, String name, byte[] image) {
this.id = id;
this.name = name;
this.image = image;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public byte[] getImage() {
return image;
}
public void setImage(byte[] image) {
this.image = image;
}
}
Create an android resource directory.
Right-click on res ⇾ and click on the android resource directory and create menu directory.
In the menu directory, create a menu resource file. Right-click on the menu and create a resource file and add search given below code.
Main_menu.xml
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto">
<item
android:title="searbar"
android:id="@+id/searchbar"
android:icon="@drawable/ic_baseline_search_24"
app:showAsAction="always"
app:actionViewClass="androidx.appcompat.widget.SearchView"/>
</menu>
Create a new Actvity
App ⇾ right click on the package name ⇾new ⇾activity ⇾empty activity.
You can name it whatever you want, here the default is named MainActivity2.java.
Logic to display data with image
private void displayData() {
sqLiteDatabase = dBmain.getReadableDatabase();
Cursor cursor = sqLiteDatabase.rawQuery("select *from " + TABLENAME + "", null);
while (cursor.moveToNext()) {
int id = cursor.getInt(0);
String name = cursor.getString(1);
byte[] image = cursor.getBlob(2);
modelArrayList.add(new Model(id, name, image));
}
//custom adapter
adapter = new Custom(this, R.layout.singledata, modelArrayList);
lv.setAdapter(adapter);
}
Declare override method for onCreateOptionMenu.
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater menuInflater = getMenuInflater();
menuInflater.inflate(R.menu.main_menu, menu);
MenuItem menuItem = menu.findItem(R.id.searchbar);
SearchView searchView = (SearchView) menuItem.getActionView();
searchView.setOnQueryTextListener(new SearchView.OnQueryTextListener() {
@Override
public boolean onQueryTextSubmit(String query) {
return false;
}
@Override
public boolean onQueryTextChange(String newText) {
adapter.getFilter().filter(newText);
return true;
}
});
return super.onCreateOptionsMenu(menu);
}
Now create an inner custom adapter class and extends BaseAdapter and implement a Filterable interface.
private class Custom extends BaseAdapter implements Filterable {
private Context context;
private int layout;
private ArrayList<Model> modelArrayList = new ArrayList<>();
private ArrayList<Model> modelArrayListFilter;
//constructor
public Custom(Context context, int layout, ArrayList<Model> modelArrayList) {
this.context = context;
this.layout = layout;
this.modelArrayList = modelArrayList;
this.modelArrayListFilter = modelArrayList;
}
//search filter
@Override
public Filter getFilter() {
Filter filter = new Filter() {
@Override
protected FilterResults performFiltering(CharSequence constraint) {
FilterResults results = new FilterResults();
ArrayList<Model> arrayListfilter = new ArrayList<>();
String searchText = constraint.toString().toLowerCase();
String[] split = searchText.split(",");
ArrayList<String> searchArr = new ArrayList<>(split.length);
for (String tsplit : split) {
String trim = tsplit.trim();
if (trim.length() > 0) {
searchArr.add(trim);
}
for (Model name : modelArrayListFilter) {
if (name.getName().toLowerCase().trim().contains(searchText)) {
arrayListfilter.add(name);
}
}
}
results.count = arrayListfilter.size();
results.values = arrayListfilter;
return results;
}
@Override
protected void publishResults(CharSequence constraint, FilterResults results) {
modelArrayList = (ArrayList<Model>) results.values;
notifyDataSetChanged();
}
};
return filter;
}
private class ViewHolder {
TextView txtname;
Button edit, delete;
ImageView imageView;
CardView cardView;
}
@Override
public int getCount() {
return modelArrayList.size();
}
@Override
public Object getItem(int position) {
return modelArrayList.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
//getting view
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder = new ViewHolder();
LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
convertView = inflater.inflate(layout, null);
//find id
holder.txtname = convertView.findViewById(R.id.txtname);
holder.imageView = convertView.findViewById(R.id.imageview);
holder.edit = convertView.findViewById(R.id.btn_edit);
holder.delete = convertView.findViewById(R.id.btn_delete);
holder.cardView = convertView.findViewById(R.id.cardview);
convertView.setTag(holder);
//random bg color
Random random = new Random();
holder.cardView.setCardBackgroundColor(Color.argb(255, random.nextInt(256), random.nextInt(255), random.nextInt(255)));
// get position of each data
Model model = modelArrayList.get(position);
//set text in textview
holder.txtname.setText(model.getName());
//set image in imageview
byte[] image = model.getImage();
Bitmap bitmap = BitmapFactory.decodeByteArray(image, 0, image.length);
holder.imageView.setImageBitmap(bitmap);
// edit buttons to edit data
holder.edit.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Bundle data = new Bundle();
data.putInt("id", model.getId());
data.putString("name", model.getName());
data.putByteArray("avatar", model.getImage());
//send data in main activity
Intent intent = new Intent(MainActivity2.this, MainActivity.class);
intent.putExtra("userdata", data);
startActivity(intent);
}
});
//delete data
holder.delete.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
sqLiteDatabase = dBmain.getReadableDatabase();
long recdelete = sqLiteDatabase.delete(TABLENAME, "id=" + model.getId(), null);
if (recdelete != -1) {
Snackbar.make(v, "record deleted=" + model.getId() + "\n" + model.getName(), Snackbar.LENGTH_LONG).show();
//for remove from current position
modelArrayList.remove(position);
notifyDataSetChanged();
}
}
});
return convertView;
}
}
Given below full code of MainActivity2.java
MainActivity2.java
package com.example.myapplication;
import androidx.appcompat.app.AppCompatActivity;
import androidx.cardview.widget.CardView;
import android.content.Context;
import android.content.Intent;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.Color;
import android.os.Bundle;
import android.view.Display;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.MenuInflater;
import android.view.MenuItem;
import android.view.View;
import android.view.ViewGroup;
import android.widget.AbsListView;
import android.widget.BaseAdapter;
import android.widget.Button;
import android.widget.Filter;
import android.widget.Filterable;
import android.widget.ImageView;
import android.widget.ListView;
import androidx.appcompat.widget.SearchView;
import android.widget.TextView;
import com.google.android.material.snackbar.Snackbar;
import java.util.ArrayList;
import java.util.Random;
import static com.example.myapplication.DBmain.TABLENAME;
public class MainActivity2 extends AppCompatActivity {
DBmain dBmain;
SQLiteDatabase sqLiteDatabase;
ListView lv;
ArrayList<Model> modelArrayList = new ArrayList<>();
Custom adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main2);
dBmain = new DBmain(this);
findid();
displayData();
}
//displaydata
private void displayData() {
sqLiteDatabase = dBmain.getReadableDatabase();
Cursor cursor = sqLiteDatabase.rawQuery("select *from " + TABLENAME + "", null);
while (cursor.moveToNext()) {
int id = cursor.getInt(0);
String name = cursor.getString(1);
byte[] image = cursor.getBlob(2);
modelArrayList.add(new Model(id, name, image));
}
//custom adapter
adapter = new Custom(this, R.layout.singledata, modelArrayList);
lv.setAdapter(adapter);
}
private void findid() {
lv = findViewById(R.id.lv);
}
//search filte
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater menuInflater = getMenuInflater();
menuInflater.inflate(R.menu.main_menu, menu);
MenuItem menuItem = menu.findItem(R.id.searchbar);
SearchView searchView = (SearchView) menuItem.getActionView();
searchView.setOnQueryTextListener(new SearchView.OnQueryTextListener() {
@Override
public boolean onQueryTextSubmit(String query) {
return false;
}
@Override
public boolean onQueryTextChange(String newText) {
adapter.getFilter().filter(newText);
return true;
}
});
return super.onCreateOptionsMenu(menu);
}
//inner class custom adapter
private class Custom extends BaseAdapter implements Filterable {
private Context context;
private int layout;
private ArrayList<Model> modelArrayList = new ArrayList<>();
private ArrayList<Model> modelArrayListFilter;
//constructor
public Custom(Context context, int layout, ArrayList<Model> modelArrayList) {
this.context = context;
this.layout = layout;
this.modelArrayList = modelArrayList;
this.modelArrayListFilter = modelArrayList;
}
//search filter
@Override
public Filter getFilter() {
Filter filter = new Filter() {
@Override
protected FilterResults performFiltering(CharSequence constraint) {
FilterResults results = new FilterResults();
ArrayList<Model> arrayListfilter = new ArrayList<>();
String searchText = constraint.toString().toLowerCase();
String[] split = searchText.split(",");
ArrayList<String> searchArr = new ArrayList<>(split.length);
for (String tsplit : split) {
String trim = tsplit.trim();
if (trim.length() > 0) {
searchArr.add(trim);
}
for (Model name : modelArrayListFilter) {
if (name.getName().toLowerCase().trim().contains(searchText)) {
arrayListfilter.add(name);
}
}
}
results.count = arrayListfilter.size();
results.values = arrayListfilter;
return results;
}
@Override
protected void publishResults(CharSequence constraint, FilterResults results) {
modelArrayList = (ArrayList<Model>) results.values;
notifyDataSetChanged();
}
};
return filter;
}
private class ViewHolder {
TextView txtname;
Button edit, delete;
ImageView imageView;
CardView cardView;
}
@Override
public int getCount() {
return modelArrayList.size();
}
@Override
public Object getItem(int position) {
return modelArrayList.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
//getting view
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder = new ViewHolder();
LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
convertView = inflater.inflate(layout, null);
//find id
holder.txtname = convertView.findViewById(R.id.txtname);
holder.imageView = convertView.findViewById(R.id.imageview);
holder.edit = convertView.findViewById(R.id.btn_edit);
holder.delete = convertView.findViewById(R.id.btn_delete);
holder.cardView = convertView.findViewById(R.id.cardview);
convertView.setTag(holder);
//random bg color
Random random = new Random();
holder.cardView.setCardBackgroundColor(Color.argb(255, random.nextInt(256), random.nextInt(255), random.nextInt(255)));
// get position of each data
Model model = modelArrayList.get(position);
//set text in textview
holder.txtname.setText(model.getName());
//set image in imageview
byte[] image = model.getImage();
Bitmap bitmap = BitmapFactory.decodeByteArray(image, 0, image.length);
holder.imageView.setImageBitmap(bitmap);
// edit buttons to edit data
holder.edit.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Bundle data = new Bundle();
data.putInt("id", model.getId());
data.putString("name", model.getName());
data.putByteArray("avatar", model.getImage());
//send data in main activity
Intent intent = new Intent(MainActivity2.this, MainActivity.class);
intent.putExtra("userdata", data);
startActivity(intent);
}
});
//delete data
holder.delete.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
sqLiteDatabase = dBmain.getReadableDatabase();
long recdelete = sqLiteDatabase.delete(TABLENAME, "id=" + model.getId(), null);
if (recdelete != -1) {
Snackbar.make(v, "record deleted=" + model.getId() + "\n" + model.getName(), Snackbar.LENGTH_LONG).show();
//for remove from current position
modelArrayList.remove(position);
notifyDataSetChanged();
}
}
});
return convertView;
}
}
}
Go to activity_main2.xml and add ListView.
Res⇾Layout⇾activity_main.xml
Add blow code in activity_main.xml.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity2">
<ListView
android:id="@+id/lv"
android:layout_width="match_parent"
android:layout_height="wrap_content"></ListView>
</LinearLayout>
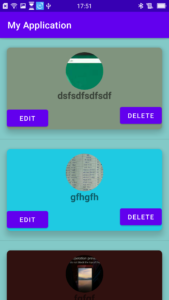
Create an XML layout resource file for a single data view.
You can see the below the code for a single data view.
singledata.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.cardview.widget.CardView xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/cardview"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:cardCornerRadius="12dp"
app:cardElevation="8dp"
app:cardPreventCornerOverlap="true"
app:cardUseCompatPadding="true">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<de.hdodenhof.circleimageview.CircleImageView
android:id="@+id/imageview"
android:layout_width="80sp"
android:layout_height="80sp"
android:layout_centerHorizontal="true"
android:layout_marginTop="10dp"
android:src="@drawable/ic_launcher_background" />
<TextView
android:id="@+id/txtname"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/imageview"
android:layout_centerInParent="true"
android:text="@string/app_name"
android:textFontWeight="400"
android:textSize="20dp"
android:textStyle="bold" />
<Button
android:id="@+id/btn_edit"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/txtname"
android:text="Edit" />
<Button
android:id="@+id/btn_delete"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/imageview"
android:layout_alignParentEnd="true"
android:layout_marginTop="20dp"
android:layout_marginEnd="-3dp"
android:text="Delete" />
</RelativeLayout>
</androidx.cardview.widget.CardView>
Now run your project.
If you want round image then add “hdodenhof Circleimageview” dependency.
Go to build.gradle and add below library
implementation 'de.hdodenhof:circleimageview:3.0.1'
Replace ImageView to add circle imageview in singledata.xml
<de.hdodenhof.circleimageview.CircleImageView
android:id="@+id/imageview"
android:layout_width="80sp"
android:layout_height="80sp"
android:layout_centerHorizontal="true"
android:layout_marginTop="10dp"
android:src="@drawable/ic_launcher_background" />
Now run the project and see the output.
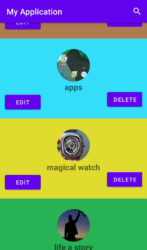