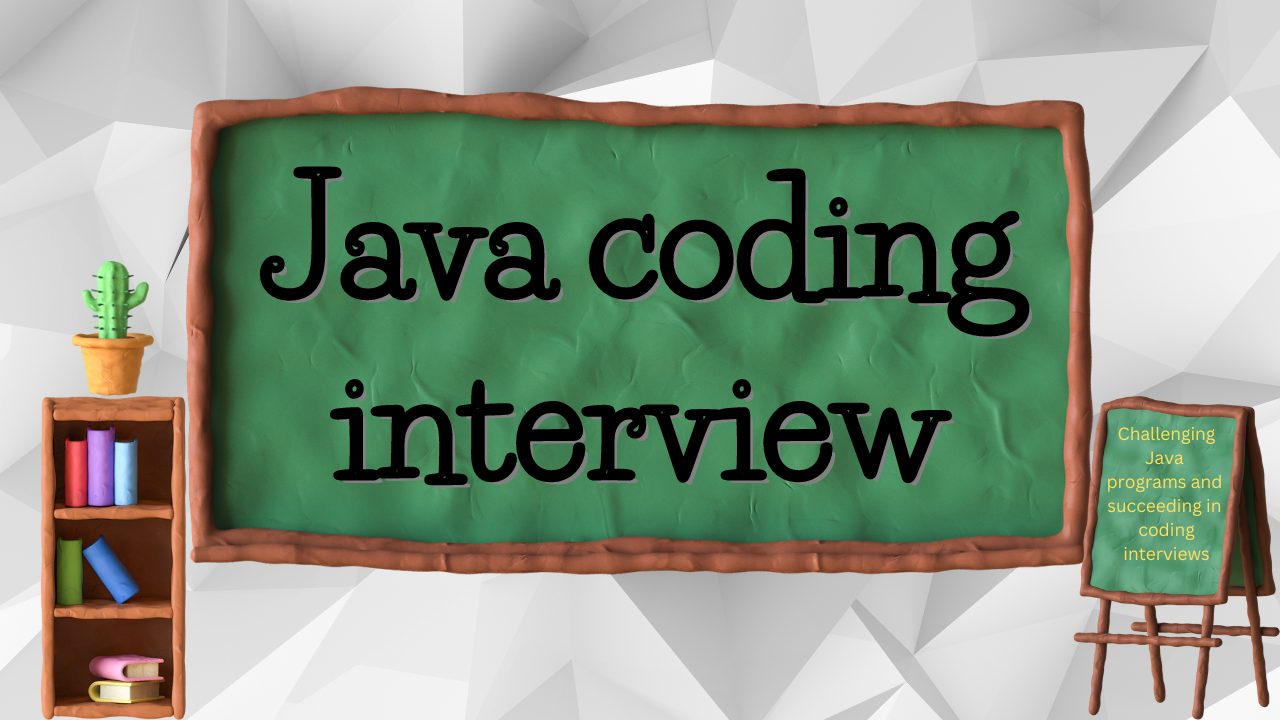
Welcome to Part-1 of our Challenging Java Programs series! In this section, we’ll be preparing a range of Java coding program examples that are commonly asked in interviews. Whether you’re a beginner or an experienced Java developer, our aim is to provide you with the knowledge and skills needed to ace your next interview. These programs require different types of solutions, and in the following section, we’ll provide you with step-by-step guidance on how to approach each one. By the end of this series, you’ll have the confidence and expertise to tackle any Java coding challenge with ease.
Write a program that calculates the sum of all even numbers between 1 and a given number
import java.util.Scanner;
public class javatechypid {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter a number: ");
int num = input.nextInt();
int sum = 0;
for (int i = 2; i <= num; i += 2) {
sum += i;
}
System.out.println("The sum of even numbers between 1 and " + num + " is " + sum + ".");
}
}
How to Run Java program in command prompt ?
Learn how to run a Java program in command prompt with our step-by-step guide
We will be using Notepad to perform Java examples so that anyone can easily run them using the command prompt (cmd).
You can run a Java program in the command prompt using the following steps:
Step 1: Open a command prompt window.
Step 2 : Navigate to the directory where your Java program is located using the “cd” command. For example, if your program is located in a folder named “java” on the desktop, you can navigate to it using the command:
cd C:\Users\YourUsername\Desktop\java
Step 3: compile the program using the “javac” command followed by the name of your Java file. For example, here program file is named “javatechypid.java”, you can compile it using the command:
javac javatechypid.java
Step 4: When program compiles successfully, you will see a new file generated in the same directory with the name “javatechypid.class”. This file contains the compiled bytecode for your Java program.
Step 5: Finally, to run your program, use the “java” command followed by the name of the class containing the main method. For example, if your main method is located in a class named “javatechypid”, you can run your program using the command:
java javatechypid
Make sure that your Java installation is configured correctly and the system PATH variable is set to include the Java bin directory so that you can run Java commands from any directory in the command prompt.
The output of the program above displays the results of the executed code.
Output:
Enter a number: 10
The sum of even numbers between 1 and 10 is 30.
Explanation of above java code:
what is ‘import java.util.Scanner’?
This line imports the Scanner class from the java.util package, which provides methods for reading user input.
what is public class javatechypid {?
This line declares a public class named javatechypid
.
what is public static void main(String[] args) {?
This line declares the main method of the program, which is the entry point for execution.
what is Scanner input = new Scanner(System.in);?
This line creates a new Scanner object named input
that reads user input from the command line.
What is System.out.print(“Enter a number: “);?
This line displays a message to the user, prompting them to enter a number.
What is int num = input.nextInt();?
This line reads an integer input from the user using the Scanner object input
and stores it in the variable num
.
What is int sum = 0; ?
This line declares and initializes a variable named sum
to 0.
What is for (int i = 2; i <= num; i += 2) {?
This line starts a for loop that iterates over all even numbers between 2 and the input number num
.
What is sum += i;?
This line adds each even number to the variable sum
.
What is System.out.println(“The sum of even numbers between 1 and ” + num + ” is ” + sum + “.”);?
This line displays the result of the calculation to the user, using the System.out.println()
method to print a message containing the input number num
and the sum of all even numbers between 2 and num
.
Overall, this program demonstrates how to use the Scanner class to read user input from the command line and how to use a for loop to iterate over a range of numbers and perform a calculation.