Well, we have already discussed “Send Data and Generate Downloadable PDF File” in the previous tutorial, but that tutorial saves the PDF file and opens it automatically once it is downloaded.
We saved the file with only one name, if you create a new input data and download it as PDF the old PDF replace a new PDF which means you can create a new PDF using this app When you download the new PDF, you will find that the old one with the same name PDF file replaces a new PDF file.
The solution to this problem is a different name according to date and time wise download Linear Layout to PDF file. When you save your PDF file, save it by date and time so that you can download unlimited PDFs, but can’t open automatically because it saved different names.
What is included in this tutorial?
We will cover the following topics in the tutorial.
- How to generate XML layout in PDF file?
- How to generate PDF file with different name?
- How to generate PDF file and save to date and time wise.
- Send data from one activity to another and generate a downloadable PDF file.
- How to create PDF file and save it with different name?
We will solve all the above questions in this tutorial.
Although we have already covered this topic but this tutorial has been updated.
So friends, let’s start creating PDF files.
How to generate layout to PDF file and save by date and time?
Step: 1
Create a new project.
Step 2:
Go to activity_main.xml.
App ⇾res⇾layout.
We will add imageview, textview and button to view the data.
The complete code of activity_main.xml is given below.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<ImageView
android:layout_marginLeft="@dimen/mlt"
android:layout_marginRight="@dimen/mrt"
android:layout_width="100dp"
android:layout_height="100dp"
android:src="@mipmap/ic_launcher"
android:id="@+id/avatar"
android:layout_gravity="center"/>
<EditText
android:layout_marginLeft="@dimen/mlt"
android:layout_marginRight="@dimen/mrt"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/fname"
android:inputType="text"
android:hint="First Name"/>
<EditText
android:layout_marginLeft="@dimen/mlt"
android:layout_marginRight="@dimen/mrt"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/lname"
android:inputType="text"
android:hint="Last Name"/>
<EditText
android:layout_marginLeft="@dimen/mlt"
android:layout_marginRight="@dimen/mrt"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/email"
android:inputType="textEmailAddress"
android:hint="Email Id"/>
<EditText
android:layout_marginLeft="@dimen/mlt"
android:layout_marginRight="@dimen/mrt"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/mobile"
android:inputType="number"
android:hint="Mobile No."/>
<EditText
android:layout_marginLeft="@dimen/mlt"
android:layout_marginRight="@dimen/mrt"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/age"
android:inputType="number"
android:hint="Age"/>
<Button
android:layout_marginLeft="@dimen/mlt"
android:layout_marginRight="@dimen/mrt"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/view"
android:text="view"/>
</LinearLayout>
Step 3:
We need some permission to write and read data.
Open AndroidManifest.xml
App⇾manifests⇾AndroidManifest.xml
Add following dependencies in AndroidManifest.xml
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
Step 4:
Open MainActivity.java and creating some method like
findId() for id casting,
private void findId() {
avatar=(ImageView)findViewById(R.id.avatar);
fname=(EditText)findViewById(R.id.fname);
lname=(EditText)findViewById(R.id.lname);
email=(EditText)findViewById(R.id.email);
mobile=(EditText)findViewById(R.id.mobile);
age=(EditText)findViewById(R.id.age);
view=(Button)findViewById(R.id.view);
}
pickImage() for pick image from gallery and display in imageview in android.
private void pickImage() {
avatar.setOnClickListener(new View.OnClickListener() {
@RequiresApi(api = Build.VERSION_CODES.M)
@Override
public void onClick(View v) {
int avatar=0;
if (avatar==0){
if (!checkStoragePermission()){
requestStoragePermission();
}else{
pickFromGallery();
}
}
}
});
}
pickFromGallery()for pick image from gallery and show in imageview
private void pickFromGallery() {
Intent intent=new Intent();
intent.setType("image/jpg/png");
intent.setAction(Intent.ACTION_GET_CONTENT);
startActivityForResult(Intent.createChooser(intent,"Pick Image"),101);
}
displayData() When click on button go to other activity and display data.
private void displayData() {
view.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent=new Intent(MainActivity.this,MainActivity2.class);
intent.putExtra("avatar",imageViewToByte());
intent.putExtra("fname",fname.getText().toString());
intent.putExtra("lname",lname.getText().toString());
intent.putExtra("email",email.getText().toString());
intent.putExtra("mobile",mobile.getText().toString());
intent.putExtra("age",age.getText().toString());
startActivity(intent);
}
});
}
The complete code of MainActivity.java is given below.
MainActivity.java
package com.example.layoutpdf;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.annotation.RequiresApi;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.content.ContextCompat;
import android.Manifest;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.graphics.Bitmap;
import android.graphics.drawable.BitmapDrawable;
import android.net.Uri;
import android.os.Build;
import android.os.Bundle;
import android.provider.MediaStore;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.Toast;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
public class MainActivity extends AppCompatActivity {
ImageView avatar;
EditText fname,lname,email,mobile,age;
Button view;
public static final int REQUEST_STORAGE=101;
String storagePermission[];
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//create methods
findId();
storagePermission=new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE};
pickImage();
displayData();
}
private void displayData() {
view.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent=new Intent(MainActivity.this,MainActivity2.class);
intent.putExtra("avatar",imageViewToByte());
intent.putExtra("fname",fname.getText().toString());
intent.putExtra("lname",lname.getText().toString());
intent.putExtra("email",email.getText().toString());
intent.putExtra("mobile",mobile.getText().toString());
intent.putExtra("age",age.getText().toString());
startActivity(intent);
}
});
}
private byte[] imageViewToByte() {
Bitmap bitmap=((BitmapDrawable)avatar.getDrawable()).getBitmap();
ByteArrayOutputStream byteArrayOutputStream=new ByteArrayOutputStream();
bitmap.compress(Bitmap.CompressFormat.JPEG,80,byteArrayOutputStream);
byte[]bytes=byteArrayOutputStream.toByteArray();
return bytes;
}
private void pickImage() {
avatar.setOnClickListener(new View.OnClickListener() {
@RequiresApi(api = Build.VERSION_CODES.M)
@Override
public void onClick(View v) {
int avatar=0;
if (avatar==0){
if (!checkStoragePermission()){
requestStoragePermission();
}else{
pickFromGallery();
}
}
}
});
}
private void pickFromGallery() {
Intent intent=new Intent();
intent.setType("image/jpg/png");
intent.setAction(Intent.ACTION_GET_CONTENT);
startActivityForResult(Intent.createChooser(intent,"Pick Image"),101);
}
@RequiresApi(api = Build.VERSION_CODES.M)
private void requestStoragePermission() {
requestPermissions(storagePermission,REQUEST_STORAGE);
}
private boolean checkStoragePermission() {
boolean result= ContextCompat.checkSelfPermission(this,Manifest.permission.WRITE_EXTERNAL_STORAGE)==(PackageManager.PERMISSION_GRANTED);
return result;
}
private void findId() {
avatar=(ImageView)findViewById(R.id.avatar);
fname=(EditText)findViewById(R.id.fname);
lname=(EditText)findViewById(R.id.lname);
email=(EditText)findViewById(R.id.email);
mobile=(EditText)findViewById(R.id.mobile);
age=(EditText)findViewById(R.id.age);
view=(Button)findViewById(R.id.view);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode==101&&resultCode==RESULT_OK){
Uri uri=data.getData();
Bitmap bitmap=null;
try {
bitmap= MediaStore.Images.Media.getBitmap(getContentResolver(),uri);
}catch (IOException e){
e.printStackTrace();
}
avatar.setImageBitmap(bitmap);
}
}
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
switch (requestCode){
case REQUEST_STORAGE:{
if (grantResults.length>0){
boolean result=grantResults[0]==PackageManager.PERMISSION_GRANTED;
if (result){
pickFromGallery();
}else{
Toast.makeText(this, "Please enable storage permission", Toast.LENGTH_SHORT).show();
}
}
}
break;
}
}
}
Step 5:
Now we want a new activity to show the data and create a PDF file.
Create a new empty Activity.
App⇾java⇾right click on package name⇾new ⇾Activity⇾Empty Activity
You can name it whatever you want, the default name given is MainActivity2.java
Step 6:
Go to the activity_main2.xml
Create a view data layout file.
The complete code of activity_main2.xml is given below.
activity_main2.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity2">
<ImageButton
android:background="@null"
android:layout_width="50dp"
android:layout_height="50dp"
android:src="@drawable/ic_baseline_save_alt_24"
android:id="@+id/save"
android:layout_centerHorizontal="true"
android:layout_alignParentBottom="true"
android:layout_marginBottom="20dp"
android:elevation="18dp"/>
<LinearLayout
android:background="@color/teal_200"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:id="@+id/lld">
<LinearLayout
android:background="@drawable/frame"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:layout_marginRight="25dp"
android:layout_marginLeft="25dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Personal Detail"
android:textStyle="bold"
android:textSize="24dp"
android:textColor="@color/white"
android:gravity="center"
android:id="@+id/textview"
android:layout_marginBottom="25dp"
android:layout_marginTop="25dp"/>
<ImageView
android:layout_centerHorizontal="true"
android:layout_marginLeft="20dp"
android:layout_width="100dp"
android:layout_height="100dp"
android:background="@mipmap/ic_launcher_round"
android:layout_below="@+id/textview"
android:id="@+id/avatarview"
/>
<TextView
android:layout_marginTop="10dp"
android:textStyle="bold"
android:textSize="20dp"
android:layout_marginLeft="30dp"
android:layout_width="165dp"
android:layout_height="wrap_content"
android:layout_below="@+id/avatarview"
android:id="@+id/textview2"
android:text="First name"/>
<TextView
android:layout_marginTop="10dp"
android:textSize="20dp"
android:layout_width="165dp"
android:layout_height="wrap_content"
android:layout_below="@+id/avatarview"
android:layout_toRightOf="@+id/textview2"
android:text="fist Name"
android:textAlignment="textStart"
android:id="@+id/fnameview"/>
<TextView
android:textStyle="bold"
android:textSize="20dp"
android:layout_marginLeft="30dp"
android:layout_width="165dp"
android:layout_height="wrap_content"
android:layout_below="@+id/textview2"
android:id="@+id/textview3"
android:text="Last name"/>
<TextView
android:textSize="20dp"
android:layout_width="165dp"
android:layout_height="wrap_content"
android:layout_below="@+id/textview2"
android:layout_toRightOf="@+id/textview2"
android:text="fist Name"
android:textAlignment="textStart"
android:id="@+id/lnameview"/>
<TextView
android:textStyle="bold"
android:textSize="20dp"
android:layout_marginLeft="30dp"
android:layout_width="165dp"
android:layout_height="wrap_content"
android:layout_below="@+id/textview3"
android:id="@+id/textview4"
android:text="Email Id"/>
<TextView
android:textSize="20dp"
android:layout_width="165dp"
android:layout_height="wrap_content"
android:layout_below="@+id/textview3"
android:layout_toRightOf="@+id/textview2"
android:text="fist Name"
android:textAlignment="textStart"
android:id="@+id/emailview"/>
<TextView
android:id="@+id/textview5"
android:layout_width="157dp"
android:layout_height="33dp"
android:layout_below="@+id/textview4"
android:layout_marginLeft="30dp"
android:text="Mobile"
android:textSize="20dp"
android:textStyle="bold" />
<TextView
android:textSize="20dp"
android:layout_width="165dp"
android:layout_height="wrap_content"
android:layout_below="@+id/textview4"
android:layout_toRightOf="@+id/textview2"
android:text="fist Name"
android:textAlignment="textStart"
android:id="@+id/mobileview"/>
<TextView
android:id="@+id/textview6"
android:layout_width="165dp"
android:layout_height="wrap_content"
android:layout_below="@+id/textview5"
android:layout_marginLeft="30dp"
android:layout_marginTop="-4dp"
android:text="age"
android:textSize="20dp"
android:textStyle="bold" />
<TextView
android:textSize="20dp"
android:layout_width="165dp"
android:layout_height="wrap_content"
android:layout_below="@+id/textview5"
android:layout_toRightOf="@+id/textview2"
android:text="Age"
android:textAlignment="textStart"
android:id="@+id/ageview"/>
<TextView
android:textSize="10dp"
android:layout_centerHorizontal="true"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Power by tehypid.com"
android:layout_alignParentBottom="true"
android:layout_alignParentEnd="true"
android:layout_marginRight="35dp"
android:layout_marginBottom="20dp"/>
<ImageView
android:backgroundTint="@color/white"
android:id="@+id/logo"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_centerHorizontal="true"
android:layout_marginBottom="80dp"
android:layout_alignParentBottom="true"
android:background="@drawable/logo"/>
</RelativeLayout>
</LinearLayout>
</LinearLayout>
</RelativeLayout>
Step 7:
Go to MainActivity2.java
In the MainActivity2.java file, we will also create some methods like Mainactivity.java.
Like
findId() for id casting.
private void findId() {
avatarview = (ImageView) findViewById ( R.id.avatarview );
fnameview = (TextView) findViewById ( R.id.fnameview );
lnameview = (TextView) findViewById ( R.id.lnameview );
emailview = (TextView) findViewById ( R.id.emailview );
mobileview = (TextView) findViewById ( R.id.mobileview );
ageview = (TextView) findViewById ( R.id.ageview );
linearLayout = findViewById ( R.id.lld );
save = findViewById ( R.id.save );
}
ViewData() for view data that send by MainActivity.java.
private void viewData() {
Intent intent = getIntent ();
byte[] bytes = intent.getByteArrayExtra ( "avatar" );
Bitmap bitmap = BitmapFactory.decodeByteArray ( bytes, 0, bytes.length );
avatarview.setImageBitmap ( bitmap );
String fname = intent.getStringExtra ( "fname" );
String lname = intent.getStringExtra ( "lname" );
String email = intent.getStringExtra ( "email" );
String mobile = intent.getStringExtra ( "mobile" );
String age = intent.getStringExtra ( "age" );
//set view
fnameview.setText ( ":-" + fname.toString () );
lnameview.setText ( ":-" + lname.toString () );
emailview.setText ( ":-" + email.toString () );
mobileview.setText ( ":-" + mobile.toString () );
ageview.setText ( ":-" + age.toString () );
}
savedPDF(); for saving PDF file with different name
private void savePdf() {
save.setOnClickListener ( new View.OnClickListener () {
@Override
public void onClick(View v) {
Log.d ( "size", linearLayout.getWidth () + " " + linearLayout.getHeight () );
bitmap = loadBitmapFromView ( linearLayout, linearLayout.getWidth (), linearLayout.getHeight () );
createPdf ();
}
} );
}
createPdf() for generate downloadable a PDF file
private void createPdf() {
DisplayMetrics displayMetrics = new DisplayMetrics ();
this.getWindowManager ().getDefaultDisplay ().getMetrics ( displayMetrics );
float height = displayMetrics.heightPixels;
float width = displayMetrics.widthPixels;
int convertHeight = (int) height,
convertWidth = (int) width;
PdfDocument document = new PdfDocument ();
PdfDocument.PageInfo pageInfo = new PdfDocument.PageInfo.Builder ( convertWidth, convertHeight, 1 ).create ();
PdfDocument.Page page = document.startPage ( pageInfo );
Canvas canvas = page.getCanvas ();
Paint paint = new Paint ();
canvas.drawPaint ( paint );
bitmap = Bitmap.createScaledBitmap ( bitmap, convertWidth, convertHeight, true );
canvas.drawBitmap ( bitmap, 0, 0, null );
document.finishPage ( page );
Calendar instance = Calendar.getInstance ();
String format = new SimpleDateFormat ( "MM/dd/yyyy", Locale.getDefault () ).format ( instance.getTime () );
String format2 = new SimpleDateFormat ( "HH:mm:ss", Locale.getDefault () ).format ( instance.getTime () );
String[] split = format.split ( "/" );
String[] split2 = format2.split ( ":" );
String str = (split[0] + split[1] + split[2]) + (split2[0] + split2[1] + split2[2]) + "_course code";
String targetPdf = "sdcard/" + str + ".pdf";
File filepath = new File ( targetPdf );
try {
document.writeTo ( new FileOutputStream ( filepath ) );
} catch (IOException e) {
e.printStackTrace ();
Toast.makeText ( this, "something want wrong try again" + e.toString (), Toast.LENGTH_SHORT ).show ();
}
//close document
document.close ();
//progress bar
ProgressDialog progressDialog;
progressDialog = new ProgressDialog ( this );
progressDialog.setMessage ( "Saving..." + targetPdf );
progressDialog.show ();
new Handler ().postDelayed ( new Runnable () {
@Override
public void run() {
progressDialog.cancel ();
}
}, 5000 );
}
The complete code of MainActivity2.java is given below.
MainActivity2.java
package com.example.layoutpdf;
import androidx.appcompat.app.AppCompatActivity;
import android.app.ProgressDialog;
import android.content.ActivityNotFoundException;
import android.content.Intent;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.Canvas;
import android.graphics.Paint;
import android.graphics.pdf.PdfDocument;
import android.net.Uri;
import android.os.Bundle;
import android.os.Environment;
import android.os.Handler;
import android.util.DisplayMetrics;
import android.util.Log;
import android.view.View;
import android.widget.ImageButton;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.TextView;
import android.widget.Toast;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Locale;
import java.util.function.BiFunction;
public class MainActivity2 extends AppCompatActivity {
ImageView avatarview;
TextView fnameview, lnameview, emailview, mobileview, ageview;
LinearLayout linearLayout;
ImageButton save;
Bitmap bitmap;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate ( savedInstanceState );
setContentView ( R.layout.activity_main2 );
findId ();
viewData ();
savePdf ();
}
private void savePdf() {
save.setOnClickListener ( new View.OnClickListener () {
@Override
public void onClick(View v) {
Log.d ( "size", linearLayout.getWidth () + " " + linearLayout.getHeight () );
bitmap = loadBitmapFromView ( linearLayout, linearLayout.getWidth (), linearLayout.getHeight () );
createPdf ();
}
} );
}
private void createPdf() {
DisplayMetrics displayMetrics = new DisplayMetrics ();
this.getWindowManager ().getDefaultDisplay ().getMetrics ( displayMetrics );
float height = displayMetrics.heightPixels;
float width = displayMetrics.widthPixels;
int convertHeight = (int) height,
convertWidth = (int) width;
PdfDocument document = new PdfDocument ();
PdfDocument.PageInfo pageInfo = new PdfDocument.PageInfo.Builder ( convertWidth, convertHeight, 1 ).create ();
PdfDocument.Page page = document.startPage ( pageInfo );
Canvas canvas = page.getCanvas ();
Paint paint = new Paint ();
canvas.drawPaint ( paint );
bitmap = Bitmap.createScaledBitmap ( bitmap, convertWidth, convertHeight, true );
canvas.drawBitmap ( bitmap, 0, 0, null );
document.finishPage ( page );
Calendar instance = Calendar.getInstance ();
String format = new SimpleDateFormat ( "MM/dd/yyyy", Locale.getDefault () ).format ( instance.getTime () );
String format2 = new SimpleDateFormat ( "HH:mm:ss", Locale.getDefault () ).format ( instance.getTime () );
String[] split = format.split ( "/" );
String[] split2 = format2.split ( ":" );
String str = (split[0] + split[1] + split[2]) + (split2[0] + split2[1] + split2[2]) + "_course code";
//pdf download location
String targetPdf = "sdcard/" + str + ".pdf";
File filepath = new File ( targetPdf );
try {
document.writeTo ( new FileOutputStream ( filepath ) );
} catch (IOException e) {
e.printStackTrace ();
Toast.makeText ( this, "something want wrong try again" + e.toString (), Toast.LENGTH_SHORT ).show ();
}
//close document
document.close ();
//progress bar
ProgressDialog progressDialog;
progressDialog = new ProgressDialog ( this );
progressDialog.setMessage ( "Saving..." + targetPdf );
progressDialog.show ();
//time after cancel
new Handler ().postDelayed ( new Runnable () {
@Override
public void run() {
progressDialog.cancel ();
}
}, 5000 );
}
private Bitmap loadBitmapFromView(LinearLayout linearLayout, int width, int height) {
bitmap = Bitmap.createBitmap ( width, height, Bitmap.Config.ARGB_8888 );
Canvas canvas = new Canvas ( bitmap );
linearLayout.draw ( canvas );
return bitmap;
}
private void viewData() {
Intent intent = getIntent ();
byte[] bytes = intent.getByteArrayExtra ( "avatar" );
Bitmap bitmap = BitmapFactory.decodeByteArray ( bytes, 0, bytes.length );
avatarview.setImageBitmap ( bitmap );
String fname = intent.getStringExtra ( "fname" );
String lname = intent.getStringExtra ( "lname" );
String email = intent.getStringExtra ( "email" );
String mobile = intent.getStringExtra ( "mobile" );
String age = intent.getStringExtra ( "age" );
//set view
fnameview.setText ( ":-" + fname.toString () );
lnameview.setText ( ":-" + lname.toString () );
emailview.setText ( ":-" + email.toString () );
mobileview.setText ( ":-" + mobile.toString () );
ageview.setText ( ":-" + age.toString () );
}
private void findId() {
avatarview = (ImageView) findViewById ( R.id.avatarview );
fnameview = (TextView) findViewById ( R.id.fnameview );
lnameview = (TextView) findViewById ( R.id.lnameview );
emailview = (TextView) findViewById ( R.id.emailview );
mobileview = (TextView) findViewById ( R.id.mobileview );
ageview = (TextView) findViewById ( R.id.ageview );
linearLayout = findViewById ( R.id.lld );
save = findViewById ( R.id.save );
}
}
Now run your project and see the output
The output is as given in the following screenshot.
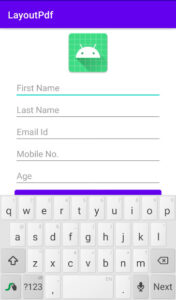
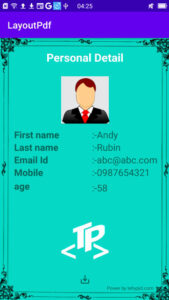