What is TimePicker ?
Time picker dialogue is display time, simply we set time in any format like 24 hours or am pm mode.
How to display TimePicker?
Here, we’ll use the edit text box, so when click on the edit Text box automatically show TimePicker dialog.
We’ll use some methods and classes to display the time picker dialog.
We’ll discuss timepicker dialog in clock mode, when you click on edit text at that time automatically display timepicker dialog and you can select a time and displaying the time that you selected.
For a dialog, we’ll use TimePickerDialog.
Let’s start with how to make a TimePicker dialog in the android studio
We’ll discuss detail step by step so below step to make time picker dialog.
Step 1:
Fired android studio
Step 2:
Select a template and click on ‘Next’.
Step 3:
Filled up project information and click on ‘finish’
Step 4:
Now your project will take some time to build Gradle.
Now, default activity MainActivity.java and activity_main.xml.
In the activity_main.xml file type the below code.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/edtdate"
android:ems="15"
android:inputType="time"
android:hint="00:00"
android:focusable="false"/>
</LinearLayout>
In XML files we’ll use simply to edit text and take id.
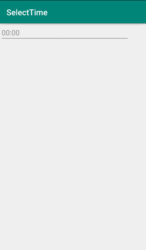
So when the user clicks on the edit text box automatically displays the time picker dialog. After selecting a time and click on ok time display on an edit text box.
So we used the edit text box to show time.
MainActivity.java
package com.example.selecttime;
import androidx.appcompat.app.AppCompatActivity;
import android.app.TimePickerDialog;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.EditText;
import android.widget.TimePicker;
import java.text.DateFormat;
import java.text.Format;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import static android.icu.util.Calendar.AM;
import static android.icu.util.Calendar.PM;
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
EditText selectTime;
private int mHour;
private int mMinute;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate ( savedInstanceState );
setContentView ( R.layout.activity_main );
selectTime = findViewById ( R.id.edtdate );
selectTime.setOnClickListener ( this );
}
@Override
public void onClick(View v) {
if (v == selectTime) {
//get time
final Calendar calendar = Calendar.getInstance ();
//launch picker dialog
final TimePickerDialog timePickerDialog = new TimePickerDialog ( this, new TimePickerDialog.OnTimeSetListener () {
@Override
public void onTimeSet(TimePicker view, int hourOfDay, int minute) {
//am pm mode
// String AM_PM;
// if (hourOfDay>=0&&hourOfDay<12){
// AM_PM=" AM";
// }else {
// AM_PM=" PM";
// }
// selectTime.setText ( hourOfDay + ":" + minute+""+AM_PM );
}
}, mHour, mMinute, false );
timePickerDialog.show ();
}
}
}
Calendar.getInstance () :
Note: uncomment the lines for am-pm mode
This method is used to get an instance of the calendar mean you can get the current time zone
calendar.get():
in this method, we’ll pass the parameter to return the value of the given calendar.
We’ll pass the parameter to return values like calendar.HOUR and calendar.MINUTE.
final Calendar calendar = Calendar.getInstance ();
mHour = calendar.get ( Calendar.HOUR );
mMinute = calendar.get ( Calendar.MINUTE );
Now, run your project.
See output.
Click on an edit text box.
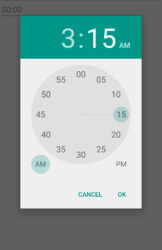
Select time.
Click on ok.
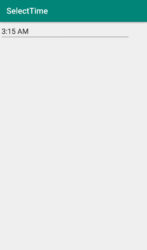
Time is set on the edit text box with am or pm mode.
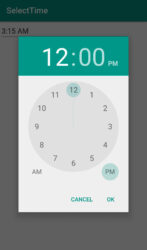
timePicker dialog pm mode ouput
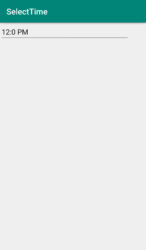