Adding menu in the action bar
Most apps have menus on the Actionbar. Additionally, you would have noticed an overflow menu on the Actionbar with different submenu items.
What new in app in android?
We will add the menu and overflow menu to the toolbar.
We will use the search icon, the eye icon and the overflow menu. Also, use the various submenu option in the overflow menu.
Clicking on the three dots open the overflow menu.
Also Read: press back button to exit android
When clicking View, another activity will open. We will create a menu folder in the res directory and add a new menu xml resource file.
Let’s build the menu on the toolbar step by step.
Step 1:
Start Android Studio and create a new project.
Step 2:
Fill all required fields and wait until gradle build is finished.
Step 3:
In this step, we’ll create a menu folder.
Right-click on the res folder ⇾ New ⇾ android recourse directory.
After making a menu, create a menu xml file to add the overflow menu and another menu on the action bar like “view” search icon etc.
As like below image
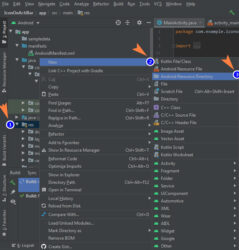
Step 4:
After creating the menu folder, add the menu resource file to it.
Right-click on Menu ⇾ New ⇾ menu resource file.
For the menu resource file, we’ll add the string value to the string file and some icons in the drawable folder.
So first, add the icon to the drawable folder that you want to add the icon to the toolbar. Then open the strings values file and add the string values as you want to create the menu.
App ⇾ Res ⇾ values ⇾ string.xml
Add the below code in the string.xml file.
strings.xml
<resources>
<string name="app_name">IconOnActiBar</string>
<string name="exit">Save and Exit</string>
<string name="protect">Protect</string>
<string name="print">Print</string>
<string name="share">Share</string>
</resources>
Next, return the menu xml file and add some menu items. In this tutorial, we will add an overflow menu, search bar and view eye menu to the action bar.
Let’s look at the menu xml file code.
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:toools="http://schemas.android.com/tools"
toools:context=".MainActivity">
<item
android:id="@+id/ac"
android:icon="@drawable/ic_remove_red_eye_black_24dp"
android:orderInCategory="1100"
android:title="menu"
app:showAsAction="always|withText" />
<item
android:id="@+id/search"
android:icon="@drawable/ic_baseline_search_24"
android:title="search"
app:showAsAction="ifRoom" />
<item
android:id="@+id/share"
android:icon="@drawable/ic_baseline_share_24"
android:title="@string/share" />
<item
android:id="@+id/print"
android:icon="@drawable/ic_baseline_print_24"
android:title="@string/print" />
<item
android:id="@+id/protect"
android:icon="@drawable/ic_baseline_security_24"
android:title="@string/protect" />
<item
android:id="@+id/exit"
android:icon="@drawable/ic_baseline_exit_to_app_24"
android:title="@string/exit" />
</menu>
In the above code, we used four menus on the overflow menu and 2 menus on the action bar menu. You can add as you like. You can also customize menu as per your app type.
ifRoom: for shoving menu on actionbar.
Lastly, the menus are ready.
Step 5:
Now, go to activity_main.xml for showing a message or image.
Below is the code for activity_main.xml.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="course code!"
android:textSize="25dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Step 6:
Go to Mainactivity. App⇾ java ⇾ package name ⇾MainActivity.java.
We’ll add the onCreateoptionsMenu() override method to show the menu icon on Actionbar.
Also Read : Create a navigation drawer
Below, code of method “onCreateOpitonsMenu.”
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater ().inflate ( R.menu.menu,menu );
return super.onCreateOptionsMenu ( menu );
}
Now, create a new override method onOptionsItemSelected for a different options like when you click on view, there will open a new activity, when you click on share icon, there will open share dialog.
Like this below code of onOptionsItemSelected.
@Override
public boolean onOptionsItemSelected(@NonNull MenuItem item) {
switch (item.getItemId()) {
case R.id.ac:
Intent intent = new Intent(this, MainActivity2.class);
startActivity(intent);
break;
case R.id.share:
//action
break;
case R.id.print:
//action
break;
case R.id.exit:
//action
break;
case R.id.protect:
//action
break;
}
return true;
}
Now let’s see the complete code of MainActivity.java class.
MainActivity.java
package com.example.icononactibar;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import android.content.ClipData;
import android.content.Intent;
import android.graphics.Color;
import android.graphics.drawable.ColorDrawable;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate ( savedInstanceState );
setContentView ( R.layout.activity_main );
getActionBar().setBackgroundDrawable(new ColorDrawable(Color.parseColor("#0ff")));
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater ().inflate ( R.menu.menu,menu );
return super.onCreateOptionsMenu ( menu );
}
@Override
public boolean onOptionsItemSelected(@NonNull MenuItem item) {
switch (item.getItemId()) {
case R.id.ac:
Intent intent = new Intent(this, MainActivity2.class);
startActivity(intent);
break;
case R.id.share:
//action
break;
case R.id.print:
//action
break;
case R.id.exit:
//action
break;
case R.id.protect:
//action
break;
}
return true;
}
}
When you click on “view or eye icon” will open a second activity, So we’ll create a new empty activity.
App ⇾ java ⇾ right click on package name ⇾ New ⇾ Activity ⇾ Empty Activity
Open MainActivity2.java class and below code for back button.
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setDisplayShowHomeEnabled(true);
Let’s see a full example.
MainActivity2.java
package com.example.icononactibar;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.Menu;
import android.widget.Toolbar;
public class MainActivity2 extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main2);
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setDisplayShowHomeEnabled(true);
}
}
Now, go to the resource file activity_main2.xml and add TextView and ImageView.
You can see the code given below.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity2">
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/courcecode"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Open AndroidManifest.xml and type the below code.
<meta-data
android:name="android.support.PARENT_ACTIVITY"
android:value=".MainActivity2" />
As a result, your app ready.
Click on Run icon.
You can see below image as output.
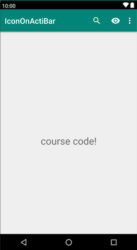
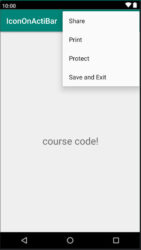
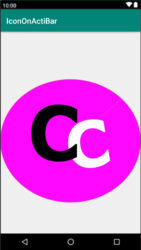
Watch on YouTube