In this tutorial, we’ll discuss TabLayout fragment tabs using Material design.
We’ll make a scroll tabs with heightened selected tab background and text.
In many apps, you have seen this functionality to fragment scroll tabs.
We’ll use single activity and different fragments so use a toolbar, TabLayout, ViewPager and fragments.
How work tabs fragment in android studio?
When you click on each tab the same page fragmentation page will open. For example, if you click on the Java tab, the Java page opens. Uses only one activity and gets a separate fragment and UI design.
Also Read: Share app link in android
We will create a simple UI design.
What is covered in this tutorial?
We’ll make 6 tabs.
We’ll extend FragmentPagerAdapter to get item position.
Change selected tab background color.
Change selected tab text color.
Let’s started step by step fragments tabs in android studio.
Step 1:
Create a new project in android studio.
Step 2:
Fill out required all fill.
Step 3:
In this step, we’ll create UI in XML file.
So navigate activity_main.xml
Res -> layout ->activity_main.xml
First of all, we’ll use androidx.appcompat.widget.Toolbar.
The toolbar widget code is given below.
<androidx.appcompat.widget.Toolbar
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/toolbar"
android:background="@color/purple_700"
android:layout_alignParentEnd="true"/>
After, we’ll use material tabs TabLayout widget.
Below code material TabLayout.
<com.google.android.material.tabs.TabLayout
app:tabSelectedTextColor="@color/black"
app:tabGravity="fill"
app:tabBackground="@drawable/tab_color"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/tablayout"
android:background="@color/teal_700"
android:layout_below="@+id/toolbar"
app:tabMode="scrollable"/>
TabLayout new attributes
app:tabSelectedTextColor="@color/black"
This attribute is used to change the color of the selected tab.
app:tabGravity="fill"
This attribute is used to space between two tabs if available.
app:tabBackground="@drawable/tab_color"
set tab background color. Before the tab background attribute, you make a drawable selector color file in xml.
So, first, we make color files in a drawable filer.
Also Read: toast message in android studio
Right-click on drawable and create a new drawable resource file.
Below code of tab_color.xml
Tab_color.xml
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@color/white" android:state_selected="true"/>
</selector>
Now implement this resource file in TabLayout.
app:tabMode="scrollable"
This attribute is used to creating a scrollable mode tab.
Now, create the ViewPager widget.
<androidx.viewpager.widget.ViewPager
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/viewpager"
android:layout_below="@+id/tablayout"/>
Full example
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<androidx.appcompat.widget.Toolbar
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/toolbar"
android:background="@color/purple_700"
android:layout_alignParentEnd="true"/>
<com.google.android.material.tabs.TabLayout
app:tabSelectedTextColor="@color/black"
app:tabGravity="fill"
app:tabBackground="@drawable/tab_color"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/tablayout"
android:background="@color/teal_700"
android:layout_below="@+id/toolbar"
app:tabMode="scrollable"/>
<androidx.viewpager.widget.ViewPager
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/viewpager"
android:layout_below="@+id/tablayout"/>
</RelativeLayout>
Step 3:
Now, create a single fragment xml resource file.
Java.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent" android:layout_height="match_parent">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="Java"
android:textSize="30dp" />
</RelativeLayout>
In this file, you use any image or text file that you want to add.
Here, every fragment resource file uses TextView.
Now create another resource file.
Python.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent" android:layout_height="match_parent">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="Phython"
android:textSize="30dp" />
</RelativeLayout>
Php.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent" android:layout_height="match_parent">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="PHP"
android:textSize="30dp" />
</RelativeLayout>
Cpp.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent" android:layout_height="match_parent">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="C++"
android:textSize="30dp" />
</RelativeLayout>
Csharp.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent" android:layout_height="match_parent">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="C#"
android:textSize="30dp" />
</RelativeLayout>
Swipt.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent" android:layout_height="match_parent">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="Swipt"
android:textSize="30dp" />
</RelativeLayout>
After creating all fragment resource files, we’ll create a java class file to extend fragments.
Step 5:
Create a java class fragment.
Java.java
package com.example.fragment_tabs;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
public class Java extends Fragment {
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
return inflater.inflate(R.layout.java,container,false);
}
}
We’ll inflate the resource files using an inflater.
After creating the same java file and extend the fragment
Python.java
package com.example.fragment_tabs;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
public class Phython extends Fragment {
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
return inflater.inflate(R.layout.python,container,false);
}
}
Php.java
package com.example.fragment_tabs;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
public class Php extends Fragment {
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
return inflater.inflate(R.layout.php,container,false);
}
}
Cpp.java
package com.example.fragment_tabs;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
public class Cpp extends Fragment {
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
return inflater.inflate(R.layout.cpp,container,false);
}
}
Csharp.java
package com.example.fragment_tabs;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
public class Csharp extends Fragment {
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
return inflater.inflate(R.layout.csharp,container,false);
}
}
Swipt.java
package com.example.fragment_tabs;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
public class Swipt extends Fragment {
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
return inflater.inflate(R.layout.swipt,container,false);
}
}
After creating all java classes, we’ll create an adapter class.
Step 6:
Create fragment adapter.
We’ll extend FragmentPagerAdapter.
After implementing the selected method. Hover mouse over red underline code and implement two methods “getItem and getCount”.
Crate variable.
Create constructer.
For the selection tab and code, we use “switch case” to get the item position.
Create variable and return variable in “getCount() method”.
The full code of the adapter.
Pageadater.java
package com.example.fragment_tabs;
import androidx.annotation.NonNull;
import androidx.fragment.app.Fragment;
import androidx.fragment.app.FragmentManager;
import androidx.fragment.app.FragmentPagerAdapter;
public class PageAdapter extends FragmentPagerAdapter {
int numCount;
public PageAdapter(@NonNull FragmentManager fm, int numCount) {
super(fm);
this.numCount = numCount;
}
public PageAdapter(@NonNull FragmentManager fm) {
super(fm);
}
@NonNull
@Override
public Fragment getItem(int position) {
switch (position){
case 0:
Java java=new Java();
return java;
case 1:
Phython phython=new Phython();
return phython;
case 2:
Php php=new Php();
return php;
case 3:
Cpp cpp=new Cpp();
return cpp;
case 4:
Csharp csharp=new Csharp();
return csharp;
case 5:
Swipt swipt=new Swipt();
return swipt;
}
return null;
}
@Override
public int getCount() {
return numCount;
}
}
Step 7:
Now, open the Main activity file.
App -> java -> packagename ->MainActivity.java
Implement “TabLayout.OnTabSelectedListener.
public class MainActivity extends AppCompatActivity implements TabLayout.OnTabSelectedListener {
Now, implement a method and create “TabLayout”, “ViewPager” and “Toolbar local variable”.
private TabLayout tabLayout;
private ViewPager viewPager;
private Toolbar toolbar;
Set toolbar before go to theme.xml file
Old android studio version go to style.xml
Change theme.
<style name="Theme.Fragment_Tabs" parent="Theme.AppCompat.NoActionBar">
After find id “TabLayout and ViewPager”
Now, set text or icon using addTab.
Tabs are creating using “newTab() method”.
setText(int) through we can set text or setIcon(int)we can set icon.
For example
package com.example.fragment_tabs;
import androidx.appcompat.app.AppCompatActivity;
import androidx.viewpager.widget.ViewPager;
import android.os.Bundle;
import android.widget.TableLayout;
import androidx.appcompat.widget.Toolbar;
import com.google.android.material.tabs.TabLayout;
public class MainActivity extends AppCompatActivity implements TabLayout.OnTabSelectedListener {
private TabLayout tabLayout;
private ViewPager viewPager;
private Toolbar toolbar;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
toolbar=(Toolbar)findViewById(R.id.toolbar);
toolbar.setTitle("Fragment TAb");
setSupportActionBar(toolbar);
tabLayout=findViewById(R.id.tablayout);
tabLayout.addTab(tabLayout.newTab().setText("JAVA"));
tabLayout.addTab(tabLayout.newTab().setText("PYTHON"));
tabLayout.addTab(tabLayout.newTab().setText("PHP"));
tabLayout.addTab(tabLayout.newTab().setText("C++"));
tabLayout.addTab(tabLayout.newTab().setText("C#"));
tabLayout.addTab(tabLayout.newTab().setText("SWIPT"));
tabLayout.setTabGravity(TabLayout.GRAVITY_CENTER);
viewPager=findViewById(R.id.viewpager);
PageAdapter adapter=new PageAdapter(getSupportFragmentManager(),tabLayout.getTabCount());
viewPager.setAdapter(adapter);
tabLayout.setOnTabSelectedListener(this);
}
@Override
public void onTabSelected(TabLayout.Tab tab) {
viewPager.setCurrentItem(tab.getPosition());
}
@Override
public void onTabUnselected(TabLayout.Tab tab) {
}
@Override
public void onTabReselected(TabLayout.Tab tab) {
}
}
Now, set adapter class.
Above all work done,
Finally, run your app.
See the output.
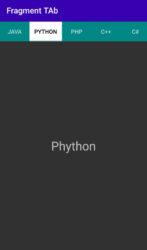
Watch on YouTube