How to display toast in android
Toast shortcut android studio
What is toast?
Toast is nothing but displaying a short message to a particular timer.
To display toast we will import toast widget, in android studio automatically import toast widget when use toast to display message.
Here, we’ll display a simple toast message. You can put custom toast with design.
When use Toast?
You can use toast when you need to displaying some method for example buttons when users click on buttons then particular display message short or long duration then we’ll use toast.
How to use toast?
You use toast simply toast class to display a simple toast message.
Method
Toast makeText (Context context, CharSequence text, in duration) display message method
Example
Toast.makeText ( MainActivity.this, “this is second toast”, Toast.LENGTH_LONG ).show ();
Toast message Example
Step 1
Open android studio and create a new project.
Step 2
Here given default MainActivity.java class and activity_main.xml file.
Step 3
We will simply use a button in XML to display the message. using the click-on button will display the short or long message, it automatically disappears after a short or long duration.
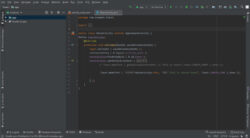
So first we’ll create a button in the activity_main.xml file
below code activity_main.xml file
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
tools:context=".MainActivity">
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/toast"
android:text="Display"/>
</LinearLayout>
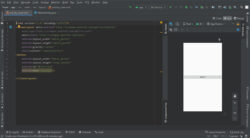
Step 4
Now, open MainActivity.java class and type below the java code.
MainActivity.java file
package com.example.toast;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
Button toastdisplay;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate ( savedInstanceState );
setContentView ( R.layout.activity_main );
toastdisplay=findViewById ( R.id.toast );
toastdisplay.setOnClickListener ( new View.OnClickListener () {
@Override
public void onClick(View v) {
// Toast.makeText ( getApplicationContext (),"this is toast",Toast.LENGTH_SHORT ).show ();
Toast.makeText ( MainActivity.this, "this is second toast", Toast.LENGTH_LONG ).show ();
}
} );
}
}
Click on the run button and run your application.
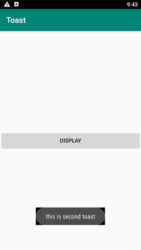
Now, we’ll discuss some methods and classes.
Here, we’ll make a simple toast message in android studio.
Toast.LENGTH_LOGN: it contains a duration of time.
LENGTH_LOGN means it displays some long duration of time.
Toast.LENGTH_SHORT: it contains the duration of time. LENGTH_SHORT means displaying messages too short a time after disappear.
What is a shortcut to toast generation?
In Android Studio, you don’t need to type complete code just type Toast and press enter, automatically the code will be completed. All you have to do is write a text message about what you want to do.