The sharing option is available in most applications whether the image gallery app or any other type of app that has a sharing option.
Using Image, you can easily share image through sharing media available on your phone.
Here we will pick an image from the gallery and view it in ImageView using GridView Image. SQLite database is a local database. We’ll used for storing and retrieving images and EditText. Server tutorials have already been created on this topic.
What is functionally in this sharing image app?
Simple and clear coding.
Sharing image with text.
View image in grid view.
If you want you can use image with crop functionality using third-party libraries then pick an image from gallery or camera which already has a tutorial about “pick an image from camera and gallery“. We can implement both codes easily.
How does it work?
We are picking images from the gallery and storing the SQLite database for demo purposes only. You can use online databases to store data. Here SQLite is used to store and retrieve data.
When clicking on the share button all type of sharing media is seen, then you can share image with text where you want.
So friends, let’s start sharing GridView Image in other apps.
How to add share image option?
Step 1
First of all, create a new project in android studio, you can name it as you want. Here is the name GridImageShare.
First, we create SQLite database to store Image and EditText data in Android.
App⇾java ⇾ right click on package name ⇾ new ⇾ java class.
Named DBmain.
Extends SQLiteOpenHelper and implements the method. Then create the database and tables.
The code below displays the DBmain.java code.
DBmain.java
package com.example.gridimageshare;
import android.content.Context;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import androidx.annotation.Nullable;
public class DBmain extends SQLiteOpenHelper {
public static final String SHARE="share.db";
public static final String IMAGETABLE="image";
public static final int VER=1;
public DBmain(@Nullable Context context) {
super(context, SHARE, null, VER);
}
@Override
public void onCreate(SQLiteDatabase db) {
String query="create table "+IMAGETABLE+"(id integer primary key, avatar blob, name text)";
db.execSQL(query);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
String query="drop table if exists "+IMAGETABLE+"";
db.execSQL(query);
}
}
Step 2
We will pick image from gallery so we need storage permission. So go to Manifests->AndroidManifest.xml and add Read External Storage and Write External Storage.
Given below is the code AndroidManifest.xml.
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.gridimageshare">
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.GridImageShare">
<activity android:name=".MainActivity2"></activity>
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Step 3
Open the default activity activity_main.xml. Here we will be using buttons for EditEext and imageview as well as for userdata input.
Activity_main.xml code is given below.
Activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<ImageView
android:id="@+id/avatar"
android:layout_width="150dp"
android:layout_height="150dp"
android:src="@mipmap/ic_launcher_round"
android:layout_gravity="center_horizontal"/>
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/name"
android:hint="Name"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<Button
android:id="@+id/submit"
android:text="submit"
android:textAllCaps="false"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"/>
<Button
android:id="@+id/display"
android:text="display"
android:textAllCaps="false"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"/>
</LinearLayout>
</LinearLayout>
Step 4
Now open MainActiviy.java
Create methods and initialize EditText, Imageview and Button.
Three methods were created in this activity findId(), insertData(), pickImage().
findId();
private void findId() {
avatar=(ImageView) findViewById(R.id.avatar);
name=(EditText)findViewById(R.id.name);
submit=(Button)findViewById(R.id.submit);
display=(Button)findViewById(R.id.display);
}
insertData();
private void insertData() {
submit.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
ContentValues cv=new ContentValues();
cv.put("avatar",imageViewToByte(avatar));
cv.put("name",name.getText().toString());
sqLiteDatabase=dBmain.getWritableDatabase();
Long recinsert=sqLiteDatabase.insert(IMAGETABLE,null,cv);
if (recinsert!=null){
Toast.makeText(MainActivity.this, "successfully inserted", Toast.LENGTH_SHORT).show();
}else{
Toast.makeText(MainActivity.this, "something wrong try again", Toast.LENGTH_SHORT).show();
}
}
});
pickImage();
private void pickImage() {
avatar.setOnClickListener(new View.OnClickListener() {
@RequiresApi(api = Build.VERSION_CODES.M)
@Override
public void onClick(View v) {
int avatar=0;
if (avatar==0){
if (!checkStoragePermission()){
requestStoragePermission();
}else{
pickFromGallery();
}
}
}
});
}
The complete code of MainActivity.java is given below.
MainActivity.java
package com.example.gridimageshare;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.annotation.RequiresApi;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.content.ContextCompat;
import android.Manifest;
import android.content.ContentValues;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.database.sqlite.SQLiteDatabase;
import android.graphics.Bitmap;
import android.graphics.drawable.BitmapDrawable;
import android.net.Uri;
import android.os.Build;
import android.os.Bundle;
import android.provider.MediaStore;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.Toast;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import static com.example.gridimageshare.DBmain.IMAGETABLE;
public class MainActivity extends AppCompatActivity {
SQLiteDatabase sqLiteDatabase;
DBmain dBmain;
ImageView avatar;
EditText name;
Button submit,display;
public static final int REQUEST_STORAGE=101;
String permissionStorage[];
Bitmap bitmap;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
dBmain=new DBmain(this);
//create method
findId();
insertData();
pickImage();
permissionStorage=new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE};
}
private void pickImage() {
avatar.setOnClickListener(new View.OnClickListener() {
@RequiresApi(api = Build.VERSION_CODES.M)
@Override
public void onClick(View v) {
int avatar=0;
if (avatar==0){
if (!checkStoragePermission()){
requestStoragePermission();
}else{
pickFromGallery();
}
}
}
});
}
private void pickFromGallery() {
Intent intent=new Intent();
intent.setType("image/*");
intent.setAction(Intent.ACTION_GET_CONTENT);
startActivityForResult(Intent.createChooser(intent,"Pick Image"),101);
}
@RequiresApi(api = Build.VERSION_CODES.M)
private void requestStoragePermission() {
requestPermissions(permissionStorage,REQUEST_STORAGE);
}
private boolean checkStoragePermission() {
boolean result= ContextCompat.checkSelfPermission(this, Manifest.permission.WRITE_EXTERNAL_STORAGE)==(PackageManager.PERMISSION_GRANTED);
return result;
}
private void insertData() {
submit.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
ContentValues cv=new ContentValues();
cv.put("avatar",imageViewToByte(avatar));
cv.put("name",name.getText().toString());
sqLiteDatabase=dBmain.getWritableDatabase();
Long recinsert=sqLiteDatabase.insert(IMAGETABLE,null,cv);
if (recinsert!=null){
Toast.makeText(MainActivity.this, "successfully inserted", Toast.LENGTH_SHORT).show();
}else{
Toast.makeText(MainActivity.this, "something wrong try again", Toast.LENGTH_SHORT).show();
}
}
});
display.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent=new Intent(MainActivity.this,MainActivity2.class);
startActivity(intent);
}
});
}
private byte[] imageViewToByte(ImageView avatar) {
Bitmap bitmap=((BitmapDrawable)avatar.getDrawable()).getBitmap();
ByteArrayOutputStream baos=new ByteArrayOutputStream();
bitmap.compress(Bitmap.CompressFormat.JPEG,80,baos);
byte[]bytes=baos.toByteArray();
return bytes;
}
private void findId() {
avatar=(ImageView) findViewById(R.id.avatar);
name=(EditText)findViewById(R.id.name);
submit=(Button)findViewById(R.id.submit);
display=(Button)findViewById(R.id.display);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode==101&&resultCode==RESULT_OK){
Uri uri=data.getData();
try {
bitmap= MediaStore.Images.Media.getBitmap(getContentResolver(),uri);
}catch (IOException e){
e.printStackTrace();
}
avatar.setImageBitmap(bitmap);
}
}
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
switch (requestCode){
case REQUEST_STORAGE:{
if (grantResults.length>0){
boolean storage_accepted=grantResults[0]==(PackageManager.PERMISSION_GRANTED);
if (storage_accepted){
pickFromGallery();
}else{
Toast.makeText(this, "please enable storage permission", Toast.LENGTH_SHORT).show();
}
}
}
break;
}
}
}
Insert data activity completed. Now we will see the data displaying activity.
Step 5
Create a Model class.
App⇾java ⇾ right click on package name ⇾ new ⇾ java class.
The code of model.java is given below.
Model.java
package com.example.gridimageshare;
public class Model {
private int id;
private byte[]avataru;
private String nameu;
//generate constructor
public Model(int id, byte[] avataru, String nameu) {
this.id = id;
this.avataru = avataru;
this.nameu = nameu;
}
//generate getter and setter method
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public byte[] getAvataru() {
return avataru;
}
public void setAvataru(byte[] avataru) {
this.avataru = avataru;
}
public String getNameu() {
return nameu;
}
public void setNameu(String nameu) {
this.nameu = nameu;
}
}
Step 6
Now, we will create single view data file in xml.
Res⇾layout ⇾new ⇾ layout resource file.
The code of single data is given below.
Singledata.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.cardview.widget.CardView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto"
app:cardElevation="6dp"
app:cardUseCompatPadding="true"
app:cardPreventCornerOverlap="true"
app:cardCornerRadius="12dp">
<RelativeLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<ImageButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_baseline_share_24"
android:layout_alignRight="@+id/avatarview"
android:elevation="18dp"
android:id="@+id/share"/>
<ImageView
android:layout_width="150dp"
android:layout_height="150dp"
android:id="@+id/avatarview"
android:scaleType="fitCenter"
android:src="@mipmap/ic_launcher_round"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/avatarview"
android:text="@string/app_name"
android:layout_centerInParent="true"
android:textStyle="bold"
android:id="@+id/nameview"
android:textSize="24dp"/>
</RelativeLayout>
</androidx.cardview.widget.CardView>
Step 7
Create Adapter class. We already know about the adapter.
App ⇾ java ⇾ right click on package name ⇾new ⇾ java class.
Named Adapter.
In onBindViewHolder we are going to add the share image option.
The code for sharing the image is given below.
holder.share.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Bitmap bitmapshare=((BitmapDrawable)holder.avatarview.getDrawable()).getBitmap();
Intent intentshare=new Intent();
intentshare.setAction(Intent.ACTION_SEND);
intentshare.setType("image/jpg/png");
ByteArrayOutputStream byteArrayOutputStream=new ByteArrayOutputStream();
bitmapshare.compress(Bitmap.CompressFormat.JPEG,100,byteArrayOutputStream);
String path= MediaStore.Images.Media.insertImage(context.getContentResolver(),bitmapshare,"Title",null);
Uri uri=Uri.parse(path);
intentshare.putExtra(Intent.EXTRA_STREAM,uri);
intentshare.putExtra(Intent.EXTRA_TEXT,"Quote:-"+model.getNameu());
intentshare.putExtra(Intent.EXTRA_SUBJECT,"Quotes");
context.startActivity(Intent.createChooser(intentshare,"Send your Image"));
}
});
The above code will use Intent, setAction(), setType(), ByteArrayOutputStream, MediaStore and putExtra to send data to other app.
setAction(Intent.ACTION_SEND); to send data.
setType(“image/jpg”); MIME type to send specify like images. If you want to send text then the MIME type should be used “text/plain”;
ByteArrayOutputStream: for written data into byte array.
MediaStore.Images.Media.insertImage(context.getContentResolver(),bitmapshare,”Title”,null); media provider for collecting media like here images.
Uri uri=Uri.parse(path); creating Uri an object.
putExtra(Intent.EXTRA_STREAM,uri); To share the path of the image you want to share.
putExtra(Intent.EXTRA_TEXT,”Quote:-“+model.getNameu());The text that is received by the model.
putExtra(Intent.EXTRA_SUBJECT,”Quotes”); Keep the subject of the message.
startActivity(Intent.createChooser(intentshare,”Send your Image”)); Send image and text target or options available to send image and text. Simple specifies a quick interface to send your data.
The code of adapter.java is given below.
Adapter.java
package com.example.gridimageshare;
import android.content.Context;
import android.content.Intent;
import android.database.sqlite.SQLiteDatabase;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.drawable.BitmapDrawable;
import android.net.Uri;
import android.provider.MediaStore;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.ImageButton;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.TextView;
import androidx.annotation.NonNull;
import androidx.recyclerview.widget.RecyclerView;
import java.io.ByteArrayOutputStream;
import java.util.ArrayList;
public class Adapter extends RecyclerView.Adapter<Adapter.ViewHolder> {
Context context;
int singledata;
ArrayList<Model>arrayList;
SQLiteDatabase sqLiteDatabase;
//generate constructor
public Adapter(Context context, int singledata, ArrayList<Model> arrayList, SQLiteDatabase sqLiteDatabase) {
this.context = context;
this.singledata = singledata;
this.arrayList = arrayList;
this.sqLiteDatabase = sqLiteDatabase;
}
@NonNull
@Override
public Adapter.ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
LayoutInflater inflater= LayoutInflater.from(context);
View view=inflater.inflate(R.layout.singledata,null);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull Adapter.ViewHolder holder, int position) {
final Model model=arrayList.get(position);
byte[]bytes=model.getAvataru();
Bitmap bitmap= BitmapFactory.decodeByteArray(bytes,0,bytes.length);
holder.avatarview.setImageBitmap(bitmap);
holder.nameview.setText(model.getNameu());
//share image
holder.share.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Bitmap bitmapshare=((BitmapDrawable)holder.avatarview.getDrawable()).getBitmap();
Intent intentshare=new Intent();
intentshare.setAction(Intent.ACTION_SEND);
intentshare.setType("image/jpg/png");
ByteArrayOutputStream byteArrayOutputStream=new ByteArrayOutputStream();
bitmapshare.compress(Bitmap.CompressFormat.JPEG,100,byteArrayOutputStream);
String path= MediaStore.Images.Media.insertImage(context.getContentResolver(),bitmapshare,"Title",null);
Uri uri=Uri.parse(path);
intentshare.putExtra(Intent.EXTRA_STREAM,uri);
intentshare.putExtra(Intent.EXTRA_TEXT,"Quote:-"+model.getNameu());
intentshare.putExtra(Intent.EXTRA_SUBJECT,"Quotes");
context.startActivity(Intent.createChooser(intentshare,"Send your Image"));
}
});
}
@Override
public int getItemCount() {
return arrayList.size();
}
public class ViewHolder extends RecyclerView.ViewHolder {
ImageView avatarview;
TextView nameview;
ImageButton share;
public ViewHolder(@NonNull View itemView) {
super(itemView);
avatarview=(ImageView)itemView.findViewById(R.id.avatarview);
nameview=(TextView)itemView.findViewById(R.id.nameview);
share=(ImageButton)itemView.findViewById(R.id.share);
}
}
}
Step 8
Create a display activity named default MainActivity2.
App ⇾java ⇾ right click on Package name ⇾activity ⇾ empty activity.
Step 9
Open activity_main2.xml and add RelativeLayotu and recyclerview.
The code of activity_main2.xml is given.
activity_main2.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity2">
<androidx.recyclerview.widget.RecyclerView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/rv"/>
</RelativeLayout>
Step 10
Now, open MainActivity2.java.
The code of MainActvity2.java is given below.
MainActivity2.java
package com.example.gridimageshare;
import androidx.appcompat.app.AppCompatActivity;
import androidx.recyclerview.widget.GridLayoutManager;
import androidx.recyclerview.widget.RecyclerView;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.os.Bundle;
import java.util.ArrayList;
import static com.example.gridimageshare.DBmain.IMAGETABLE;
public class MainActivity2 extends AppCompatActivity {
SQLiteDatabase sqLiteDatabase;
DBmain dBmain;
RecyclerView recyclerView;
Adapter myadater;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main2);
dBmain=new DBmain(this);
//create method
findId();
dispalyData();
recyclerView.setLayoutManager(new GridLayoutManager(this,3));
}
private void dispalyData() {
sqLiteDatabase=dBmain.getReadableDatabase();
Cursor cursor=sqLiteDatabase.rawQuery("select *from "+IMAGETABLE+"",null);
ArrayList<Model>arrayList=new ArrayList<>();
while (cursor.moveToNext()){
int id=cursor.getInt(0);
byte[]avatar=cursor.getBlob(1);
String name=cursor.getString(2);
arrayList.add(new Model(id,avatar,name));
}
cursor.close();
myadater=new Adapter(this,R.layout.singledata,arrayList,sqLiteDatabase);
recyclerView.setAdapter(myadater);
}
private void findId() {
recyclerView=findViewById(R.id.rv);
}
}
Now run your app and see the output. You can see the output below.
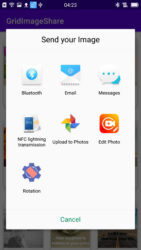
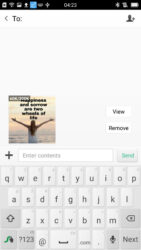