In this tutorial, we’ll create CheckBoxs with SQLite database and display CheckBox data into ListView.
We’ll use a local and open-source SQLite database for storing data into the user’s device.
SQLite is easy to set up, storing, manipulating, or retrieving persistent data from the database.
How works this CheckBox app?
It is important how it works.
Here, we’ll make such an app that store checkbox data in SQLite database and display data in ListView.
Generally, you can make multiple checkbox and store in SQLite database.
Here, two checkboxes and a two-button are used. One button is for submitting and the other button is for display the database.
We’ll use define the click handler for buttons.
When the user clicks on the “submit” button, the Button object receives an on-click event.
Clicking on the “Display” button will open another activity, where you will go to display the data.
Let’s understand with an example.
Step 1:
We’ll create a new project in android studio.
Step 2:
Fill all required fill and start android projects.
Step 3:
Now, the project is ready to create a checkbox app in android studio.
First, we need UI(user interface) so we’ll create a design view in XML file.
App -> res-> Layout-> activity_main.xml
As can see following code which is given layout with checkbox and button.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity" >
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Select your favorite Subject"
android:textSize="18dp"
android:textStyle="bold" />
<CheckBox
android:id="@+id/checkBox"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Algorithms" />
<CheckBox
android:id="@+id/checkBox2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Data Structure" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="submit" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Display" />
</LinearLayout>
</LinearLayout>
Step 4:
Now, we required a new activity to displaying data, so we’ll create a new activity.
File -> new -> activity -> Empty Activity
In the new activity, we’ll create a Listview in xml file to display data in list format.
activity_dispaly_data.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".DisplayData">
<ListView
android:id="@+id/lv"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:divider="@color/purple_200"
android:dividerHeight="1dp"
android:listSelector="@color/teal_200" />
</LinearLayout>
Step 5:
Now, we’ll create a single data resource file to show the data in ListView.
We’ll use TextView to display checkboxes data into TextView.
Right click on Layout -> Layout resource file.
Singledata.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent" android:layout_height="match_parent">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="TextView"
android:textSize="18dp"
android:textStyle="bold" />
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentEnd="true"
android:text="TextView"
android:textSize="18dp"
android:textStyle="bold" />
</RelativeLayout>
Step 6:
Finlay, UI design complete. Now create a database.
App -> java -> package name
Right-click on the package name and create a new java class.
DBmain.java
package com.pd.checkbox;
import android.content.Context;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import androidx.annotation.Nullable;
public class DBmain extends SQLiteOpenHelper {
public static final String DB="student";
public static final int VER=1;
public DBmain(@Nullable Context context) {
super(context, DB, null, VER);
}
@Override
public void onCreate(SQLiteDatabase db) {
String query="create table course(id integer primary key,sub text,sub2 text)";
db.execSQL(query);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
String drop="drop table if exists course";
db.execSQL(drop);
onCreate(db);
}
}
In the above code, we have extended helper class SQLiteOpenHelper for managing database, creation and version manage.
After, extends SQLiteOpenHelper, we have implementation some method like onCreate() and onUpgrade() methods of SQLiteOpenHelper class.
Then, we created a database and table query. Have also used drop table query.
Step7:
In this step, we’ll insert data into databases so create method insertData();
Right-click on it or hover mouser over it and create a method.
We’ll use a button click listener.
Given below, code for insert data into the database.
private void insertData() {
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
sqLiteDatabase=dBmain.getWritableDatabase();
ContentValues contentValues=new ContentValues();
if (checkBox.isChecked())
contentValues.put("sub",checkBox.getText().toString());
if (checkBox2.isChecked())
contentValues.put("sub2",checkBox2.getText().toString());
Long rec=sqLiteDatabase.insert("course",null,contentValues);
if (rec!=null){
Toast.makeText(MainActivity.this, "data inserted", Toast.LENGTH_SHORT).show();
}else{
Toast.makeText(MainActivity.this, "data not inserted", Toast.LENGTH_SHORT).show();
}
}
});
We have used two buttons in activity_main.xml layout design, one is submitted and the other is displayed. The data will show while clicking on the display button.
Button2 mean displaydata
button2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
startActivity(new Intent(MainActivity.this,DisplayData.class));
}
});
Then, we create a new method to find the ID.
private void findid() {
button=(Button)findViewById(R.id.button);
button2=(Button)findViewById(R.id.button2);
checkBox2=(CheckBox)findViewById(R.id.checkBox2);
checkBox=(CheckBox)findViewById(R.id.checkBox);
}
Now go to MainActivity.java.
MainActivity.java
package com.pd.checkbox;
import androidx.appcompat.app.AppCompatActivity;
import android.content.ContentValues;
import android.content.Intent;
import android.database.sqlite.SQLiteDatabase;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
DBmain dBmain;
SQLiteDatabase sqLiteDatabase;
Button button,button2;
CheckBox checkBox,checkBox2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
dBmain=new DBmain(MainActivity.this);
findid();
insertData();
}
private void insertData() {
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
sqLiteDatabase=dBmain.getWritableDatabase();
ContentValues contentValues=new ContentValues();
if (checkBox.isChecked())
contentValues.put("sub",checkBox.getText().toString());
if (checkBox2.isChecked())
contentValues.put("sub2",checkBox2.getText().toString());
Long rec=sqLiteDatabase.insert("course",null,contentValues);
if (rec!=null){
Toast.makeText(MainActivity.this, "data inserted", Toast.LENGTH_SHORT).show();
}else{
Toast.makeText(MainActivity.this, "data not inserted", Toast.LENGTH_SHORT).show();
}
}
});
//display data
button2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
startActivity(new Intent(MainActivity.this,DisplayData.class));
}
});
}
private void findid() {
button=(Button)findViewById(R.id.button);
button2=(Button)findViewById(R.id.button2);
checkBox2=(CheckBox)findViewById(R.id.checkBox2);
checkBox=(CheckBox)findViewById(R.id.checkBox);
}
}
In the above code, we inserted data in the SQLite database in your local device.
We used two method, findid(); and insertData();
Also used button and onClickListener event.
When the button is clicked, the data will be stored in the SQLite database.
Inserted the data into the database, now we will display the data.
Go to DisplayData.java class,
And create a method findid() same as previously created on Mainactivity.java class.
We’ll find the id by listview.
private void findid() {
listView=findViewById(R.id.lv);
}
Create a displayData method.
private void displayData() {
sqLiteDatabase=dBmain.getReadableDatabase();
Cursor cursor=sqLiteDatabase.rawQuery("select *from course",null);
if (cursor.getCount()>0){
id=new int[cursor.getCount()];
name=new String[cursor.getCount()];
name2=new String[cursor.getCount()];
int i=0;
while(cursor.moveToNext()){
id[i]=cursor.getInt(0);
name[i]=cursor.getString(1);
name2[i]=cursor.getString(2);
i++;
}
Custom custom=new Custom();//adapter
listView.setAdapter(custom);
}
}
Now, create a custom adapter
Custom custom=new Custom();
listView.setAdapter(custom);
Create class clicking on it and extends BaseAdapter and implement abstract method hover on it.
private class Custom extends BaseAdapter {
@Override
public int getCount() {
return name.length;
}
@Override
public Object getItem(int position) {
return null;
}
@Override
public long getItemId(int position) {
return 0;
}
Finally, we’ll be displaying data using layoutInflater and set the position.
@Override
public View getView(int position, View convertView, ViewGroup parent) {
TextView textView,textView1;
convertView= LayoutInflater.from(DisplayData.this).inflate(R.layout.singledata,parent,false);
textView=(TextView)convertView.findViewById(R.id.textView);
textView1=(TextView)convertView.findViewById(R.id.textView2);
textView.setText(name[position]);
textView1.setText(name2[position]);
return convertView;
}
}
See the full example of DispalyData.java
DisplayData.java
package com.pd.checkbox;
import androidx.appcompat.app.AppCompatActivity;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ListView;
import android.widget.TextView;
public class DisplayData extends AppCompatActivity {
DBmain dBmain;
SQLiteDatabase sqLiteDatabase;
ListView listView;
String[]name,name2;
int[]id;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_display_data);
dBmain=new DBmain(DisplayData.this);
findid();
displayData();
}
private void displayData() {
sqLiteDatabase=dBmain.getReadableDatabase();
Cursor cursor=sqLiteDatabase.rawQuery("select *from course",null);
if (cursor.getCount()>0){
id=new int[cursor.getCount()];
name=new String[cursor.getCount()];
name2=new String[cursor.getCount()];
int i=0;
while(cursor.moveToNext()){
id[i]=cursor.getInt(0);
name[i]=cursor.getString(1);
name2[i]=cursor.getString(2);
i++;
}
Custom custom=new Custom();
listView.setAdapter(custom);
}
}
private void findid() {
listView=findViewById(R.id.lv);
}
private class Custom extends BaseAdapter {
@Override
public int getCount() {
return name.length;
}
@Override
public Object getItem(int position) {
return null;
}
@Override
public long getItemId(int position) {
return 0;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
TextView textView,textView1;
convertView= LayoutInflater.from(DisplayData.this).inflate(R.layout.singledata,parent,false);
textView=(TextView)convertView.findViewById(R.id.textView);
textView1=(TextView)convertView.findViewById(R.id.textView2);
textView.setText(name[position]);
textView1.setText(name2[position]);
return convertView;
}
}
}
Now, run your app and see.
An output similar to the image below will be seen.
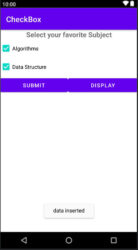
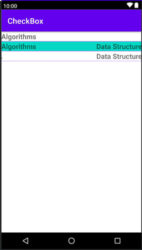
Watch on YouTube