What is the Alertdialog box?
AlertDialog is nothing but a type that alerts you before doing any action.
For example, if you want to exit the app, then you press the back button. Only then the AlertDialog is shown.
In a previous tutorial, we showed you “Press Back to Exit“. This alert dialog is very similar to the alert dialog we used in this tutorial. An alert dialog is a user graphical UI design. When the user does something wrong while showing alert.
Why use this alert dialog in these apps?
In this tutorial, we will create a simple alert dialog app in Android. Shows alert when you press the back button. If you want to exit then you press the back button then alert dialog will appear.
What is special in this alert dialog?
- This alert dialog is a simple user interface.
- AlertDialog title.
- AlertDialog icon
- Make AlertDialog box message.
- This AlertDialog in include two buttons positive(YES) and negative(NO)
- YES and NO button’s background color.
- Height and width of the AlertDialog box.
- AlertDialog background image.
How to make this type of alert dialog box in android?
Now, we come to the point.
Let’s start creating an awesome AlertDialog in android.
Step 1:
Start new android project.
Step 2:
In the activity_main.xml file, we’ll set any message. No needed but only for example.
App -> src -> main -> res -> layout -> activity_main.xml
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Power by Techypid.com"
android:textSize="16dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Now, see the main code of AlertDialog.
App -> src -> main -> java -> com.example.alertdialog -> MainActivity.java
MianActivity.java
package com.example.alertdialog;
import androidx.appcompat.app.AlertDialog;
import androidx.appcompat.app.AppCompatActivity;
import android.annotation.SuppressLint;
import android.content.DialogInterface;
import android.os.Bundle;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
@SuppressLint("ResourceAsColor")
public void onBackPressed() {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("New simple Alert dialog");
builder.setIcon(R.drawable.ic_exit_to_app_black_24dp);
builder.setMessage("Do you really want to exit?")
.setCancelable(false)
.setPositiveButton("Yes", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
finish();
}
})
.setNegativeButton("no", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
dialog.cancel();
}
});
AlertDialog alertDialog = builder.create();
alertDialog.show();
alertDialog.getButton(AlertDialog.BUTTON_POSITIVE).setBackgroundColor(getResources().getColor(R.color.green));
alertDialog.getButton(AlertDialog.BUTTON_NEGATIVE).setBackgroundColor(getResources().getColor(R.color.red));
//SET HEIGHT AND WIDTH OF ALERT DIALOG BOX
int width = (int) (getResources().getDisplayMetrics().widthPixels * 0.85);
int height = (int) (getResources().getDisplayMetrics().heightPixels * 0.30);
alertDialog.getWindow().setLayout(width, height);
alertDialog.getWindow().setBackgroundDrawableResource(R.drawable.ic_launcher_background);//ALERTDIALOG BACKGROUND IMAGE
}
}
In above code shown some methods.
Why use this method?
onBackPressed(): When the back button is pressed, the method “onBackPressed()” is called. And AlertDialog will appear.
builder.setTitle: Set the main header of your alert dialog.
Builder.setIcon: To set a small icon or logo for the alert dialog.
builder.setMessage: To set the message in AlertDialog.
.setCancellable: AlertDialog Canceled or not.
.setPositiveButton: Exit when the button is clicked. (“Yes”, new DialogInterface. onClickListener()
AlertDialog.getButton: To get two buttons “positive” and negative.
AlertDialog.getWindow(): To set background image from our drawable folder.
Now, we’ll add some colors for the positive and negative background buttons.
Open color.xml resource file. Locate app -> src -> main -> res -> values – >colors.xml.
Color.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="colorPrimary">#008577</color>
<color name="colorPrimaryDark">#00574B</color>
<color name="colorAccent">#D81B60</color>
<color name="red">#F1420A</color>
<color name="green">#07F812</color>
</resources>
Your alert dialog is complete.
Let’s see the output of the alert dialog.
The output is shown in the below image.
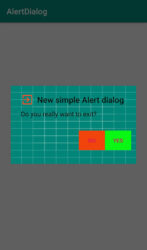
Watch on YouTube