In the previous tutorial, we inserted data with images into the MySQL database. Also, we stored images in the image folder.
Step: 1
First, we convert PHP MySQL database data to JSON format.
Guys, we are going to show the data in android RecyclerView, so first we convert the PHP data into JSON format and then will get the data using the retrofit library.
The following code demonstrates encoding MySQL data.
Select.php
<?php
require("config.php");
$query="SELECT *FROM student_data";
$result=mysqli_query($conn,$query);
$rows=array();
$folder='student_data';
$file='photo';
$url="https://androidcode99.000webhostapp.com";
$fulpath="$url/$folder/$file";
while($row=mysqli_fetch_assoc($result)){
array_push($rows,array(
'id'=>$row['id'],
'name'=>$row['name'],
'image'=>"$fulpath/".$row['image']));
}
echo json_encode($rows);
mysqli_close($conn);
?>
Our PHP data to JSON format data is ready. Now we will show data in android RecyclerView.
Step: 2
Start an android studio
Step: 3
Create a new project in Android Studio
Step: 4
Add dependencies
Open build.gradle (module:.app) and add following dependencies.
Gradle scripts ⇾ build.gradle
implementation 'com.squareup.retrofit2:retrofit:2.9.0'
implementation 'com.squareup.retrofit2:converter-gson:2.9.0'
implementation'com.squareup.picasso:picasso:2.5.2'
Step: 5
Add internet permission
Manifest ⇾AndroidManifest.xml
We will be parsing and displaying the data in Android RecyclerView, which is formatted in MySQL data to JSON. So we need to add internet permission.
<uses-permission android:name="android.permission.INTERNET"/>
Step: 6
Now, create layout design in activity_main.xml.
So open activity_main.xml and add the following code
Activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<androidx.swiperefreshlayout.widget.SwipeRefreshLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/swipeRefresh">
<androidx.recyclerview.widget.RecyclerView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/rv"/>
</androidx.swiperefreshlayout.widget.SwipeRefreshLayout>
</RelativeLayout>
Step: 7
Singleview
Now, we create singleview layout file.
Res⇾layout⇾create a new file.
The following code demonstrates singleview.xml
Singleview.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="wrap_content">
<ImageView
android:src="@drawable/ic_launcher_background"
android:layout_width="200dp"
android:layout_height="200dp"
android:layout_gravity="center"
android:id="@+id/imageview"/>
<TextView
android:textSize="25dp"
android:text="@string/app_name"
android:gravity="center"
android:layout_gravity="center"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/textview"/>
</LinearLayout>
Step: 8
Now go to the java folder and create a model class inside the package name.
Java⇾right click on the package name⇾new ⇾java class.
Create a Model named java class.
The following code demonstrates mode.java
Model.java
package com.example.phpjson;
import com.google.gson.annotations.SerializedName;
class Model {
private int id;
@SerializedName ( "name" )
private String name;
@SerializedName ( "image" )
private String image;
//generate constructor
public Model(int id, String name, String image) {
this.id = id;
this.name = name;
this.image = image;
}
//getter and setter method
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getImage() {
return image;
}
public void setImage(String image) {
this.image = image;
}
}
Step: 9
Create an interface java class.
Java⇾right click on package name⇾new⇾java class ⇾choose interface.
Add the following code in the interface class
MyApi.java
package com.example.phpjson;
import java.util.ArrayList;
import retrofit2.Call;
import retrofit2.http.GET;
interface MyApi {
@GET("select.php")
Call<ArrayList<Model>>modelArray();
}
Step: 10
Create an adapter class named MyAdapter.
Java⇾right click on class name⇾new⇾java class
Add following code in MyAdapter.java
MyAdapter.java
package com.example.phpjson;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.TextView;
import androidx.annotation.NonNull;
import androidx.recyclerview.widget.RecyclerView;
import com.squareup.picasso.Picasso;
import java.util.ArrayList;
import java.util.zip.Inflater;
class MyAdapter extends RecyclerView.Adapter<MyAdapter.ViewHolder> {
private ArrayList<Model>modelArrayList;
private Context context;
//generate constructor
public MyAdapter(ArrayList<Model> modelArrayList, Context context) {
this.modelArrayList = modelArrayList;
this.context = context;
}
@NonNull
@Override
public MyAdapter.ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view= LayoutInflater.from ( parent.getContext () ).inflate ( R.layout.singleview,parent,false );
return new ViewHolder (view);
}
@Override
public void onBindViewHolder(@NonNull MyAdapter.ViewHolder holder, int position) {
//set data
Model model=modelArrayList.get ( position );
Picasso.with ( context ).load ( model.getImage () )
.error ( R.mipmap.ic_launcher )
.into ( holder.imageView );
//for text
holder.textView.setText ( model.getName () );
}
@Override
public int getItemCount() {
return modelArrayList.size ();
}
public class ViewHolder extends RecyclerView.ViewHolder {
ImageView imageView;
TextView textView;
public ViewHolder(@NonNull View itemView) {
super ( itemView );
imageView=(ImageView)itemView.findViewById ( R.id.imageview );
textView=(TextView)itemView.findViewById ( R.id.textview );
}
}
}
Step: 11
Go to MainActivity.java
Java⇾package name⇾MainActivity.java
The following code demonstrates MainActivity.java.
MainActivity.java
package com.example.phpjson;
import androidx.appcompat.app.AppCompatActivity;
import androidx.recyclerview.widget.LinearLayoutManager;
import androidx.recyclerview.widget.RecyclerView;
import android.os.Bundle;
import android.widget.BaseAdapter;
import android.widget.Toast;
import java.util.ArrayList;
import retrofit2.Call;
import retrofit2.Callback;
import retrofit2.Response;
import retrofit2.Retrofit;
import retrofit2.converter.gson.GsonConverterFactory;
public class MainActivity extends AppCompatActivity {
MyAdapter myAdapter;
MyApi myApi;
String BaseUrl="https://androidcode99.000webhostapp.com/student_data/";
RecyclerView recyclerView;
ArrayList<Model>modelArrayList;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate ( savedInstanceState );
setContentView ( R.layout.activity_main );
modelArrayList=new ArrayList<> ();
//initialize id
recyclerView=findViewById ( R.id.rv );
displayJsonData();
}
private void displayJsonData() {
Retrofit retrofit=new Retrofit.Builder ()
.baseUrl ( BaseUrl )
.addConverterFactory ( GsonConverterFactory.create () )
.build ();
//call api class
myApi=retrofit.create ( MyApi.class );
Call<ArrayList<Model>>modelCall=myApi.modelArray ();
modelCall.enqueue ( new Callback<ArrayList<Model>> () {
@Override
public void onResponse(Call<ArrayList<Model>> call, Response<ArrayList<Model>> response) {
modelArrayList=response.body ();
for (int i=0;i<modelArrayList.size ();i++){
//set adapter
myAdapter=new MyAdapter ( modelArrayList,MainActivity.this );
//implementation layout manager
LinearLayoutManager layoutManager=new LinearLayoutManager ( MainActivity.this,RecyclerView.VERTICAL,false );
//set layout manager
recyclerView.setLayoutManager ( layoutManager );
recyclerView.setAdapter ( myAdapter );
}
}
@Override
public void onFailure(Call<ArrayList<Model>> call, Throwable t) {
//failed if not load
Toast.makeText ( MainActivity.this, "failed to load", Toast.LENGTH_SHORT ).show ();
}
} );
}
}
Now, it’s time to run your project.
You will get output like the following.
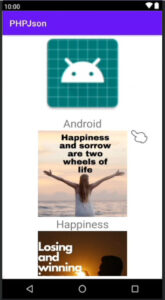
We showed you MySQL data in android RecyclerView with images.
When you upload a new image in PHP MySQL database, a new record will also show in Android RecyclerView.
Note: In this post, if you find any mistakes or bugs and errors, feel free to contact or give feedback to improve the quality. Great if you give your feedback about this tutorial and other tutorials.