In this Laravel tutorial, we will cover the basics of the project structure for a Laravel application. Laravel’s structure includes various directories and files with different names, each having a specific purpose for file management. Let’s dive in and gain a better understanding of Laravel’s project structure.
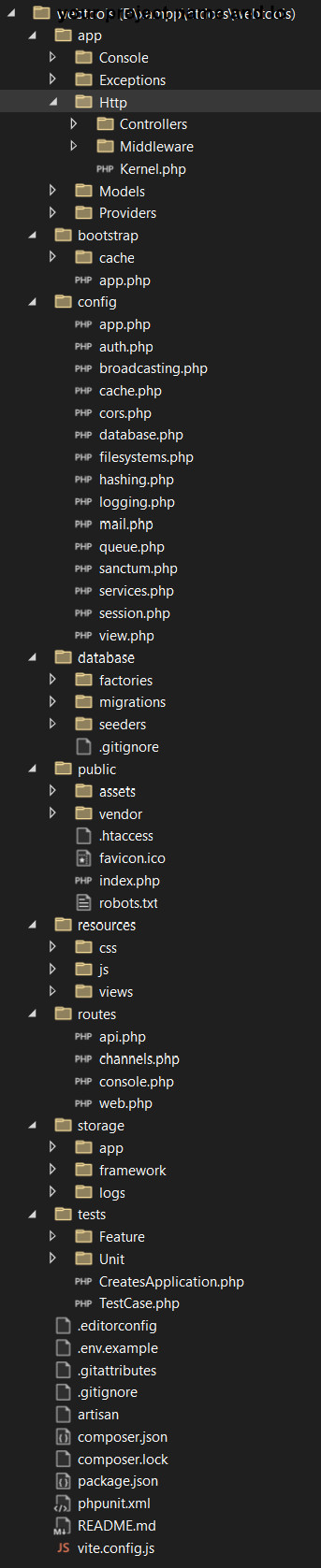
Table of Contents
LaravelProject
As you can see in the above Image, the first directory is the project directory which you named yourself.
app
This folder contains the core code of your Laravel application. It is where you will define your models, controllers, service providers, and other application logic.
The app directory is where you put most of the code that makes up your Laravel application. It contains subdirectories and files that organize your application’s code into logical units.
Console: This directory contains the code for your application’s Artisan commands. Artisan is Laravel’s command-line interface, and it provides a convenient way to perform common tasks like migrating the database or generating code.
Exceptions: This directory contains the code for your application’s exception handlers. Exception handlers are responsible for handling any exceptions that are thrown by your application, and they provide a way to gracefully handle errors and display informative error messages to users.
Http: This directory contains most of the code for your application’s HTTP interface. It contains subdirectories for Controllers, Middleware, and Requests, as well as a Kernel class that handles HTTP requests.
- Controllers: This directory contains your application’s controller classes. Controllers are responsible for handling incoming HTTP requests and returning responses to the client.
- Middleware: This directory contains your application’s middleware classes, as I mentioned in my previous message. Middleware are classes that sit between the incoming HTTP request and the application’s response, and they can modify the request or response, or perform additional processing before or after the request is handled by your application.
- Kernel: This class is the entry point for incoming HTTP requests to your application. It handles the middleware stack and routes the request to the appropriate controller or closure. The Kernel class defines the middleware stack, which is a series of middleware classes that are applied to incoming requests in the order they are defined.
Models: This directory contains the code for your application’s data models. Models define the structure and behavior of the data that your application stores, and they provide a way to interact with the database in a convenient and consistent way.
Providers: This directory contains the code for your application’s service providers. Service providers are responsible for registering the various services and components that your application uses, such as database connections, email services, or third-party libraries.
bootstrap
This folder contains the files that bootstrap your application, including the app.php file and the cache folder
app.php: This file loads the Laravel framework and creates an instance of the application, which is then used to handle incoming HTTP requests.
cache: This directory contains cached files that are generated by Laravel to speed up the application’s bootstrapping process.
config
This folder contains all the configuration files for your application, including settings for your database, mail, file systems, and more.
app.php: This file contains basic configuration settings for your application, such as the application name, environment, timezone, and debug mode.
auth.php: This file contains the configuration settings for your application’s authentication system, such as the default guard driver, password reset settings, and user provider.
broadcasting.php: This file contains the configuration settings for your application’s broadcasting system, such as the default broadcaster, connection settings, and authentication driver.
cache.php: This file contains the configuration settings for your application’s caching system, such as the default cache driver, cache expiration times, and cache prefixes.
cors.php: This file contains the configuration settings for Cross-Origin Resource Sharing (CORS), which is a mechanism that allows resources on a web page to be requested from another domain.
database.php: This file contains the configuration settings for your application’s database connection, such as the database driver, host, port, and credentials.
filesystems.php: This file contains the configuration settings for your application’s file storage system, such as the default disk driver, disk URLs, and disk permissions.
hashing.php: This file contains the configuration settings for your application’s password hashing system, such as the default algorithm and cost.
logging.php: This file contains the configuration settings for your application’s logging system, such as the log channels, log levels, and log file locations.
mail.php: This file contains the configuration settings for your application’s mail system, such as the mail driver, host, port, and credentials.
queue.php: This file contains the configuration settings for your application’s queue system, such as the default queue driver, connection settings, and job retry settings.
sanctum.php: This file contains the configuration settings for Laravel Sanctum, which is a lightweight package for API authentication using tokens.
services.php: This file contains the configuration settings for third-party services that your application might use, such as social media authentication providers or payment gateways.
session.php: This file contains the configuration settings for your application’s session management, such as the default session driver, session lifetime, and session encryption.
view.php: This file contains the configuration settings for your application’s view system, such as the default view engine, view paths, and compiled view file locations.
database
This folder contains everything related to your database, including factories, migrations and seeders.
factories: This directory contains model factory files, which are used to generate fake data for your application’s models. This is especially useful for testing and development, as it allows you to easily create large amounts of realistic test data.
migrations: This directory contains database migration files, which are used to modify the database schema in a structured way. Migrations allow you to version your database schema and easily roll back changes if needed.
seeders: This directory contains database seeder files, which are used to populate the database with dummy data for testing and development purposes.
.gitignore: The .gitignore file is a configuration file that is used by Git to determine which files and directories should be ignored when committing changes to a repository.
public
This folder contains the public-facing files for your application, such as CSS, JavaScript, and images. The index.php file in this folder is the entry point for your application.
assets: The assets directory typically contains static files such as images, CSS stylesheets, and JavaScript files that are used to render the user interface of a web application.
vendor: the vendor directory in a Laravel application contains all of the dependencies that are installed by Composer, which is a package manager for PHP. When you install a package using Composer, it will download the package’s source code and any dependencies it requires, and place them in the vendor directory.
.htaccess or nginx.conf: These are configuration files that are used to configure the web server (Apache or Nginx) that is serving the application. These files can be used to redirect traffic, set environment variables, and perform other server-side tasks.
favicon.ico: This is a small icon file that is displayed in the browser tab for the application.
index.php: This file is the main entry point for the application, and is responsible for bootstrapping the Laravel framework and routing incoming requests to the appropriate controller method.
robots.txt: this is a text file that is used to provide instructions to web robots or crawlers about which pages or files on a website they are allowed or not allowed to crawl or index.
resources
This folder contains all the non-PHP files that your application needs, such as views, JavaScript, CSS file.
css: It contains all the CSS files that are used to style the web pages of the application.
js: This directory contains all the JavaScript files used by the application.
views: This directory contains all the Blade templates used to render the user interface of the application.
routes
In routes directory, you define the routes for your Laravel application. Routes are the entry points to your application, and they specify which controller method should be called when a particular URL is accessed.
The default routes directory in a Laravel application contains two files:
web.php: This file contains routes for web interfaces of your application, such as pages that users can access in their browsers. These routes typically use the HTTP verbs GET, POST, PUT, and DELETE.
api.php: This file contains routes for the API interface of your application, such as endpoints that other applications or services can use to interact with your application. These routes typically use the HTTP verbs GET, POST, PUT, PATCH, and DELETE.
You can also see two other files in the routes directory of a Laravel application:
console.php: This file contains routes for Laravel’s command-line interface (CLI) commands. These routes allow you to define custom commands that can be run from the terminal, and specify the actions that should be taken when those commands are executed.
channels.php: This file contains routes for Laravel’s broadcasting feature, which allows you to broadcast events and messages to subscribed clients. These routes typically use Laravel’s WebSocket-based broadcasting protocol, but can also support other broadcasting technologies like Pusher.
storage
This folder contains files that are generated by your application, such as app, framework and logs, files.
app: This directory is used to store files that are specific to the application, such as user-generated files, session files, and uploaded files.
framework: This directory is used to store files that are generated by the Laravel framework, such as cache files, session files, and view files.
logs: This directory is used to store the log files generated by the application. By default, Laravel logs all errors and warnings that occur in the application, as well as any messages that are explicitly logged by the developer using the Log facade.
tests
This folder contains all the tests for your application, including unit tests, feature tests, and integration tests.
Feature: This directory is used to store feature tests, which test the application’s behavior from a user’s perspective.
These tests typically simulate HTTP requests to the application and assert the expected responses.
Unit: This directory is used to store unit tests, which test individual units of code in isolation.
These tests typically exercise a single method or function and assert the expected behavior.
Apart from these subdirectories, you can also find other files and directories such as:
createApplication.php: This file is used to create a new instance of the application for testing purposes.
TestCase.php: This file is the base test case for the application. It’s used to set up the testing environment and provide helper methods for writing tests.
.editorconfig : This file is a configuration file that defines the coding style and formatting for a project.
.env.example: This file is an example configuration file that defines environment variables used by the application.
.gitattributes, .gitignore: These files are used to define Git configuration and ignore files that should not be tracked by Git.
artisan: This file is the command-line interface for Laravel. It’s used to run commands, such as migrations and tests.
composer.json, composer.lock: These files define the dependencies and packages required by the application.
package.json: This file defines the JavaScript dependencies required by the application.
phpunit.xml: This file is the configuration file for PHPUnit, the testing framework used by Laravel.
README.md: This file contains the documentation for the application.
vite.config.js: This file is used to configure Vite, the build tool used by Laravel to compile JavaScript and CSS assets.
Overall, the basic structure of a Laravel application provides a well-organized framework for building web applications, with clear separation of concerns between the different components of the application.